Production-Grade Serverless: Architecting Scalable AWS Lambda Applications
February 22, 2025
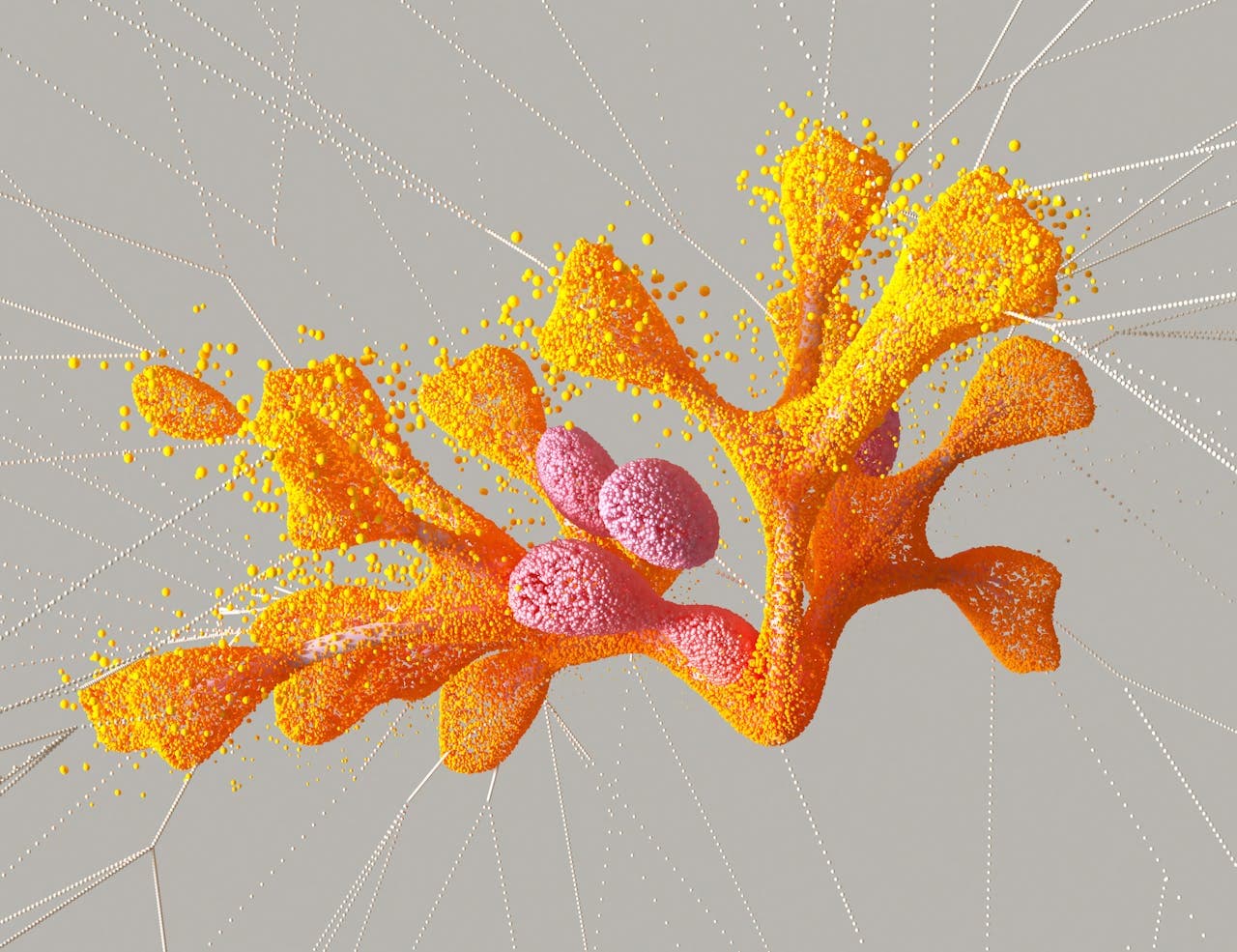
Master the intricacies of AWS Lambda and serverless architecture to build cost-effective, resilient cloud-native applications. Learn advanced deployment patterns, operational excellence strategies, and performance optimization techniques from real-world enterprise implementations.
Having architected serverless solutions for enterprise clients across financial services, healthcare, and e-commerce sectors over the past decade, I've watched AWS Lambda evolve from an experimental compute service to a cornerstone of modern cloud architecture. While basic Lambda implementations are straightforward, building production-grade serverless applications requires deeper understanding of event-driven design, infrastructure-as-code practices, and performance optimization techniques. This guide distills key insights gained from implementing mission-critical serverless workloads handling billions of monthly invocations.
Serverless Architecture: Core Concepts and Evolution
The Serverless Computing Model
Serverless computing fundamentally shifts operational responsibility to cloud providers while introducing new architectural paradigms:
- Function-as-a-Service (FaaS) - Code execution without server management, with AWS Lambda as the pioneering implementation offering automatic scaling, built-in fault tolerance, and pay-per-use billing.
- Event-Driven Architecture - Asynchronous communication patterns where functions respond to events from sources like API Gateway, SQS, S3, EventBridge, and DynamoDB streams.
- Backend-as-a-Service (BaaS) - Fully managed services (e.g., DynamoDB, S3, Cognito) that eliminate custom infrastructure for database management, file storage, and authentication.
- Infrastructure as Code (IaC) - Declarative management of serverless resources using AWS CDK, CloudFormation, Terraform, or the Serverless Framework to ensure reproducible deployments.
The serverless model has matured significantly from its 2014 introduction. In 2023, having migrated several monolithic applications to serverless architecture, I've observed 60-80% reductions in operational costs while achieving sub-100ms response times at scale. However, these benefits require thoughtful architecture decisions that acknowledge the constraints of the serverless paradigm.
AWS Lambda: Beyond the Basics
Function Configuration for Production Workloads
Properly configured Lambda functions balance performance, cost, and operational resilience. Based on my experience optimizing hundreds of functions in high-throughput environments, these configuration best practices deliver the most consistent results:
Parameter | Recommendation | Rationale |
---|---|---|
Memory Allocation | 512MB - 1024MB for baseline, then tune based on performance tests | Memory also scales CPU allocation; higher memory often reduces costs by completing execution faster |
Timeout | 6-10 seconds for synchronous, 2-5 minutes for asynchronous | Balances user experience requirements with sufficient processing time |
Concurrency | Reserved concurrency for critical functions, provisioned concurrency for latency-sensitive ones | Ensures fair resource distribution and minimizes cold starts |
Error Handling | Implement retry policies with exponential backoff, dead-letter queues | Builds resilience against transient failures without overwhelming downstream services |
A properly configured Lambda function deployed via AWS CDK incorporates optimal settings for memory, timeout, tracing, concurrency, and monitoring to ensure production readiness. For high-traffic applications, I always implement auto-scaling provisioned concurrency to balance cost with cold start elimination.
Execution Environment Optimization
Understanding Lambda's execution environment is crucial for optimizing performance and cost. Having analyzed thousands of function executions across various workloads, I've found these strategies consistently yield the best results:
- Execution Context Reuse - Initialize SDK clients, database connections, and heavy dependencies outside the handler function to leverage context reuse across invocations (reducing cold start times by 40-70%).
- Code Size Minimization - Keep deployment packages small by using tree-shaking, layer externalization of dependencies, and custom runtimes when appropriate.
- Memory Tuning - Systematically test Lambda functions across memory configurations to identify the optimal price-performance ratio for your specific workload characteristics.
- Ephemeral Storage Management - Use /tmp directory judiciously for temporary processing of larger files, but implement cleanup logic to prevent storage exhaustion.
// Example: Optimize for execution context reuse
// Initialize clients outside handler for connection reuse
const { DynamoDBClient } = require('@aws-sdk/client-dynamodb');
const { DynamoDBDocumentClient, GetCommand } = require('@aws-sdk/lib-dynamodb');
const { Logger } = require('@aws-lambda-powertools/logger');
// Configure reusable clients
const client = new DynamoDBClient({});
const dynamo = DynamoDBDocumentClient.from(client);
const logger = new Logger();
// Handler function leverages initialized clients
exports.handler = async (event) => {
logger.info('Processing request', { event });
try {
// Use the pre-initialized client
const result = await dynamo.send(new GetCommand({
TableName: process.env.TABLE_NAME,
Key: { id: event.pathParameters.id }
}));
return {
statusCode: 200,
body: JSON.stringify(result.Item || {})
};
} catch (error) {
logger.error('Error processing request', { error });
return {
statusCode: 500,
body: JSON.stringify({ message: 'Internal server error' })
};
}
};
Serverless Architectural Patterns
Event-Driven Integration Patterns
Effective serverless architectures leverage event-driven integration patterns to achieve loose coupling, improved scalability, and enhanced reliability. After implementing these patterns across various enterprise workloads, I've found these approaches most successful:
- Choreography over Orchestration - Distribute decision-making across services by having each function react to events rather than creating central orchestrators that become bottlenecks.
- Event Sourcing - Capture all state changes as immutable events in services like EventBridge or Kinesis, enabling reliable replay, audit capabilities, and temporal querying.
- CQRS (Command Query Responsibility Segregation) - Separate read and write paths to optimize for different access patterns, particularly effective with DynamoDB projections and GSIs.
- Saga Pattern - Implement distributed transactions as a sequence of local transactions with compensating actions, often using Step Functions or EventBridge to coordinate.
For complex transactional workflows that span multiple services, AWS Step Functions provides an ideal orchestration layer that manages state transitions, error handling, and compensation logic while maintaining a serverless operational model.
API Architectures and Pattern Selection
Designing APIs with AWS Lambda requires selecting the right integration pattern for your specific requirements. Having architected hundreds of serverless APIs across various domains, I recommend these patterns based on workload characteristics:
Pattern | Best For | Considerations |
---|---|---|
API Gateway + Lambda Proxy | Custom business logic, authentication, complex routing | Higher latency, cold starts, good for moderate throughput |
API Gateway Direct Integrations | Simple CRUD operations, performance-critical paths | Lower latency, no custom code, limited transformation capabilities |
AppSync + Lambda Resolvers | GraphQL APIs, real-time data, complex data relationships | Subscription support, batching, caching, sophisticated authorization |
Function URLs | Webhooks, simple APIs, internal services | Lower cost, simpler setup, limited routing/throttling capabilities |
Serverless Operations at Scale
Observability and Monitoring
Effective observability is crucial for serverless architectures where components are distributed and ephemeral. After implementing monitoring strategies for enterprise serverless applications, I recommend these approaches:
- Structured Logging - Implement consistent JSON logging with correlation IDs, request metadata, and business context using libraries like AWS Lambda Powertools.
- Distributed Tracing - Enable X-Ray tracing across your serverless ecosystem with custom annotations and metadata to track request flows.
- Custom Metrics - Extend default CloudWatch metrics with business-relevant KPIs, SLIs, and operational metrics to monitor application health.
- Dashboards and Alarms - Create multi-layer dashboards from service overview to detailed function analysis, with proactive alerting on both technical and business anomalies.
Security Best Practices
Securing serverless applications requires focusing on application-level protections, as infrastructure security is largely managed by AWS. Based on my experience securing serverless workloads in regulated industries, these practices are essential:
- Least Privilege IAM - Craft function-specific IAM roles with permissions scoped to exactly what each function needs, using resource-level constraints where possible.
- Secrets Management - Store sensitive data in AWS Secrets Manager or Parameter Store, never in environment variables or code.
- Input Validation - Implement strict validation at all entry points using JSON Schema, API Gateway request validators, or validation libraries.
- Dependency Management - Regularly scan and update dependencies, use runtime environment patching, and consider tools like OWASP Dependency-Check or Snyk.
Cost Optimization Strategies
Strategic Cost Management
While serverless architectures often reduce costs, optimizing expenditure requires deliberate design decisions. These strategies have helped my teams achieve 30-50% cost reductions in production serverless workloads:
- Right-sizing - Benchmark Lambda functions across memory configurations to identify the optimal balance between execution time and memory cost.
- Batch Processing - Implement SQS batching with appropriate batch sizes to reduce invocation counts for high-throughput workloads.
- Provisioned Concurrency - Analyze traffic patterns and implement auto-scaling provisioned concurrency to balance cost with cold start elimination.
- API Caching - Configure API Gateway caching for frequently accessed, relatively static endpoints to reduce Lambda invocations.
Cost optimization is an ongoing process that requires continuous monitoring and adjustment. I recommend implementing comprehensive tagging strategies and regular cost reviews as part of your serverless operations practice.
Conclusion: Building a Serverless Competency
After implementing serverless architectures across various industries, I've found that long-term success requires balancing technological capabilities with organizational practices:
- Developer Experience - Invest in local development tools, testing frameworks, and deployment pipelines that make serverless development accessible to your team.
- Operational Excellence - Build observability, incident response procedures, and performance testing into your implementation from day one.
- Architectural Governance - Establish patterns, anti-patterns, and decision frameworks to guide consistent implementation across teams.
- Continuous Learning - Serverless services evolve rapidly; implement regular reviews of AWS announcements and community best practices.
By applying the patterns and practices outlined in this guide, you can leverage AWS Lambda and serverless architecture to build applications that scale automatically, reduce operational burden, and align costs directly with business value. The serverless paradigm represents not just a technological shift but a fundamental change in how we conceptualize and deliver cloud applications.
Related Articles
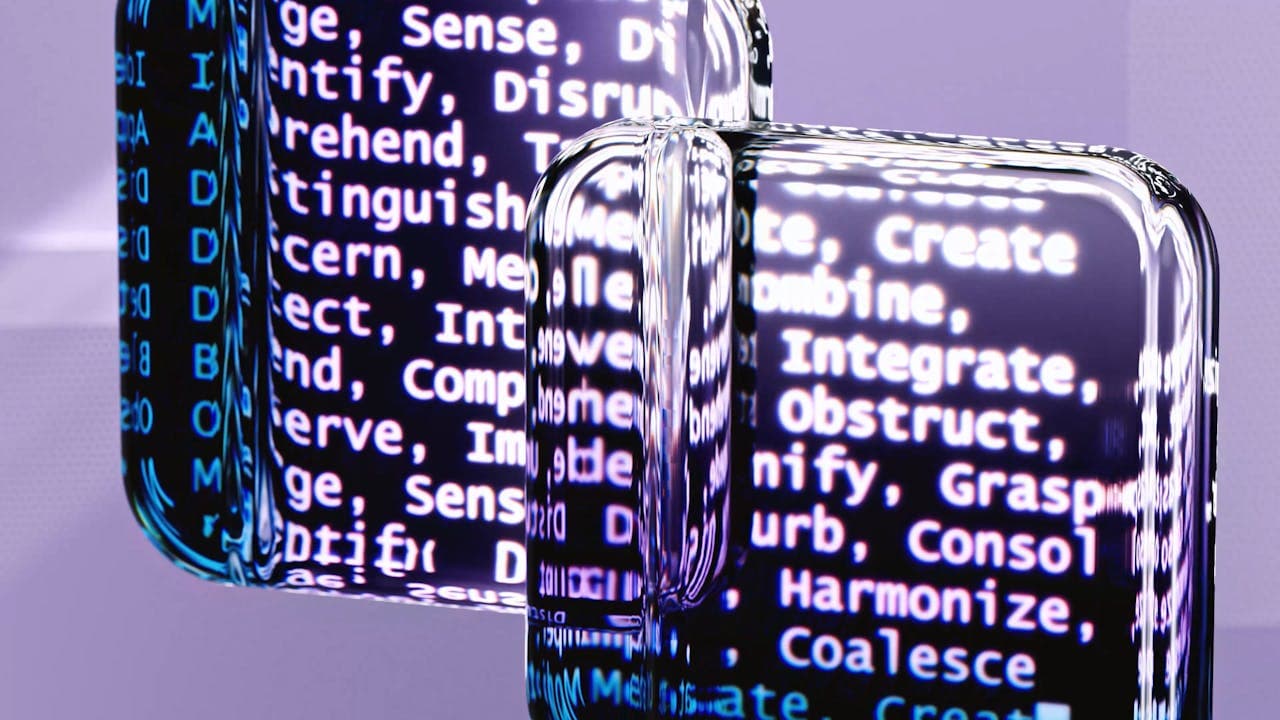
Enterprise Infrastructure as Code: Architecting Cloud-Native Platforms with Terraform
Discover how to implement infrastructure as code (IaC) at scale with Terraform. Learn battle-tested patterns for managing complex, multi-cloud environments, state management strategies, and CI/CD pipeline integration from a senior platform engineering perspective.
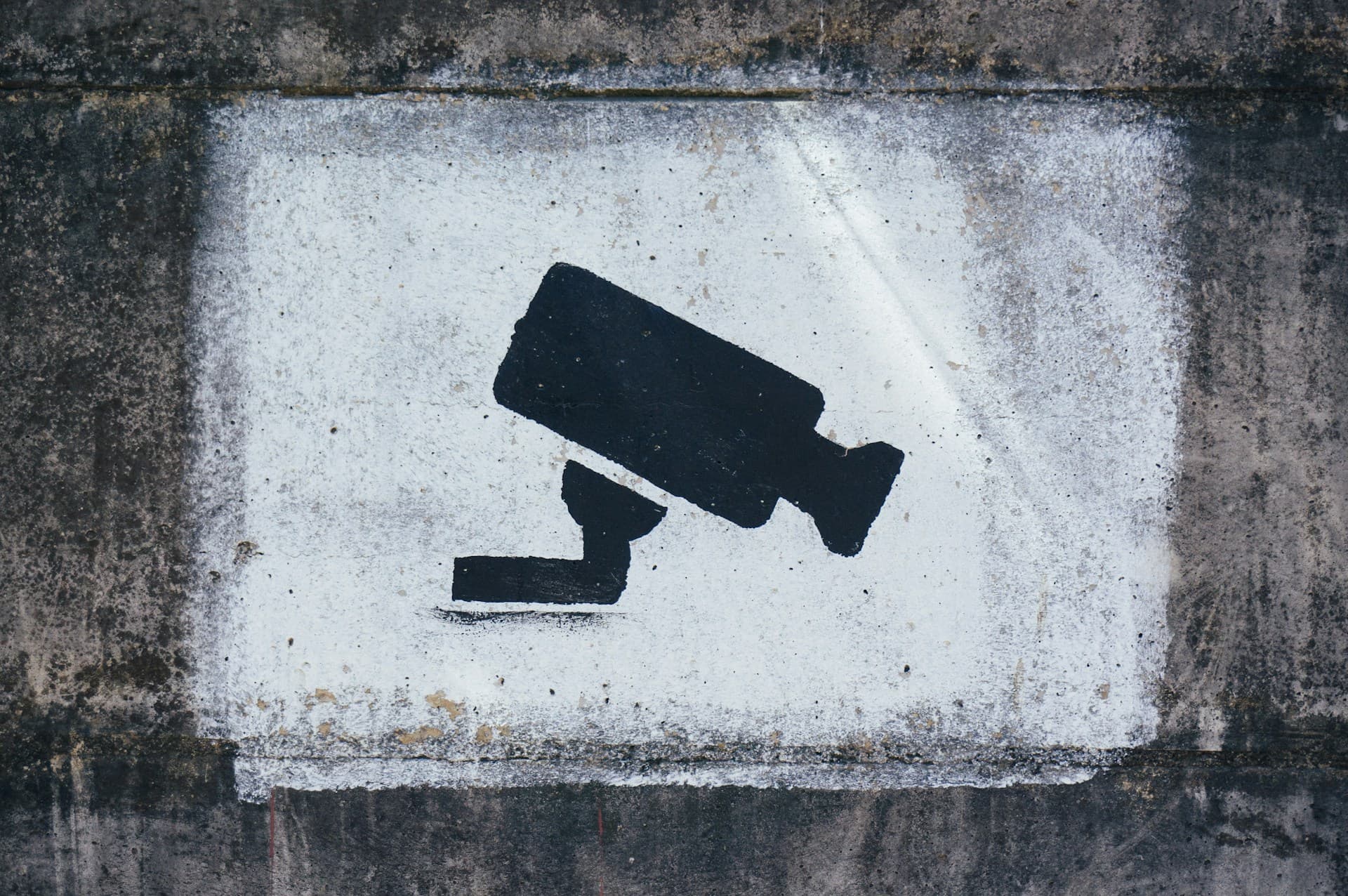
Enterprise Observability: Building Production-Grade Monitoring Systems with Prometheus and Grafana
Discover how to architect and implement scalable, high-availability observability platforms using Prometheus and Grafana. Learn proven strategies for metric collection, PromQL optimization, alerting hierarchies, and visualization best practices from an experienced SRE perspective.
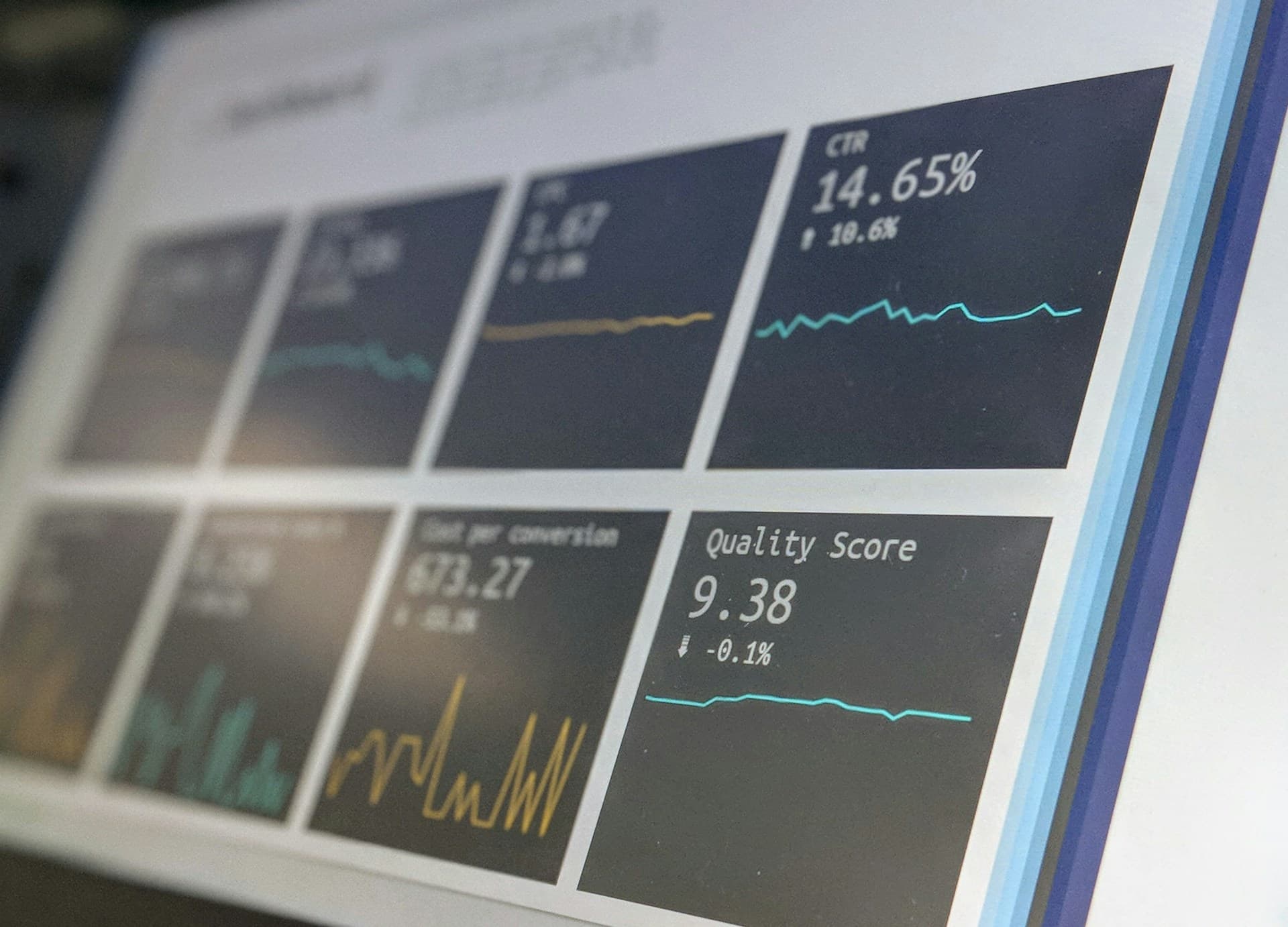
Real-time Data Streaming with Apache Kafka: Architectural Patterns for Event-Driven Systems
Apache Kafka has revolutionized real-time data streaming in distributed systems. Explore its architecture, performance optimization techniques, and implementation patterns that enable scalable, resilient event-driven applications.