Real-time Data Streaming with Apache Kafka: Architectural Patterns for Event-Driven Systems
February 8, 2025
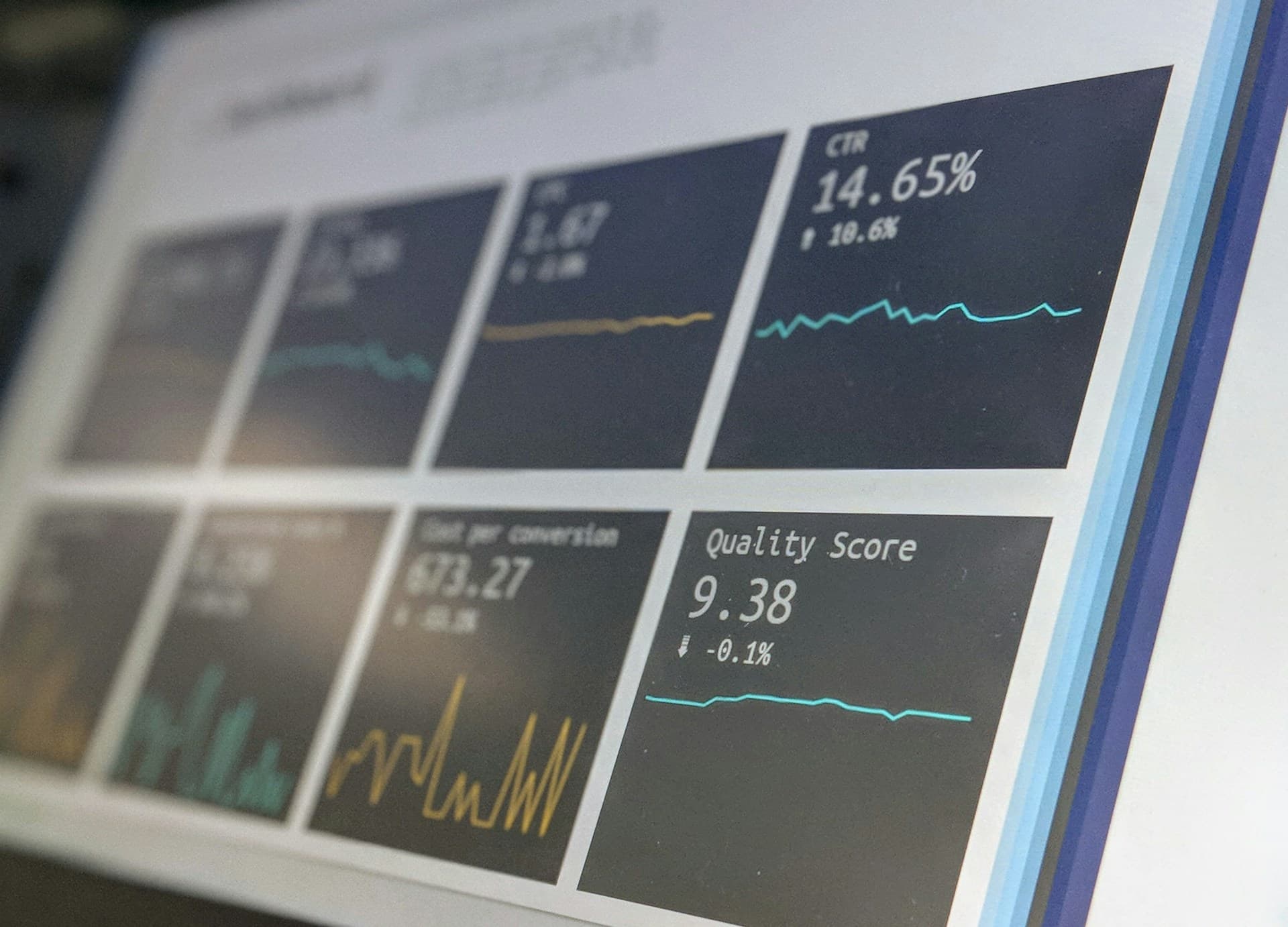
Apache Kafka has revolutionized real-time data streaming in distributed systems. Explore its architecture, performance optimization techniques, and implementation patterns that enable scalable, resilient event-driven applications.
After architecting event-driven systems with Apache Kafka for nearly a decade, I've witnessed its evolution from a specialized messaging queue to a comprehensive event streaming platform that forms the backbone of modern data infrastructures. Organizations across industries are leveraging Kafka to process trillions of events daily, enabling real-time analytics, microservices communication, and data integration at unprecedented scale.
The Evolution of Data Processing Architectures
To appreciate Kafka's significance, we must understand the evolution of data processing paradigms:
Paradigm | Processing Model | Primary Use Cases | Limitations |
---|---|---|---|
Batch Processing | Periodic processing of accumulated data | Reporting, ETL, data warehousing | High latency, stale insights |
Message Queues | Point-to-point message delivery | Task distribution, workload decoupling | Limited scalability, single-consumer model |
Pub/Sub | Multi-consumer broadcasting | Notifications, real-time updates | Limited persistence, no replay capability |
Stream Processing | Continuous processing of unbounded data | Real-time analytics, event-driven applications | Complexity in stateful processing, ordering guarantees |
Kafka emerged as a response to limitations in both traditional batch processing systems and earlier messaging technologies. By combining persistent storage, publish-subscribe semantics, and horizontal scalability, Kafka created a new category of technology that supports both real-time event streaming and historical data access.
Apache Kafka's Core Architecture
At its foundation, Kafka's architecture consists of several key components that work together to provide scalable, fault-tolerant data streaming capabilities:

Apache Kafka's distributed architecture with brokers, topics, partitions, and consumer groups
Topics and Partitions
Topics are the fundamental organizational unit in Kafka, representing a particular stream of data. Each topic is divided into partitions, which are the basic unit of parallelism and scalability:
- Partitions: Ordered, immutable sequence of records that are continually appended
- Partition Distribution: Spread across brokers for parallel processing and fault tolerance
- Partition Offsets: Unique sequential IDs assigned to messages within a partition
When designing your topic structure, consider these partition sizing guidelines:
# Partition calculation formula
partitions = max(throughput_requirements / partition_throughput, consumer_parallelism)
# Example for 1GB/sec throughput with 100MB/sec per partition and 20 parallel consumers
partitions = max(1000MB/sec / 100MB/sec, 20) = max(10, 20) = 20
Brokers and Zookeeper
Kafka's distributed nature relies on a cluster of brokers coordinated by ZooKeeper (or more recently, KRaft in Kafka 3.0+):
- Brokers: Servers that store topic partitions and handle produce/consume requests
- Controller: Special broker responsible for administrative operations
- ZooKeeper/KRaft: Manages cluster state, broker health, and configuration
- Replication: Each partition has multiple replicas for fault tolerance
Producers and Consumers
Kafka's client APIs enable applications to produce and consume data:
- Producers: Write data to topics, with control over partition assignment
- Consumers: Read data from topics, maintaining their position via offsets
- Consumer Groups: Collection of consumers that collectively process topic data
- Rebalancing: Dynamic redistribution of partitions when consumers join/leave
Production-Grade Kafka Implementation Patterns
Based on my experience implementing Kafka in enterprises across financial services, e-commerce, and telecommunications sectors, here are the patterns that lead to successful deployments:
1. Multi-Cluster Architectures
Organizations operating at scale typically implement multiple Kafka clusters for isolation and resilience:
- Regional Clusters: Separate clusters per geographic region to minimize latency
- Domain Separation: Dedicated clusters for different business domains or data classifications
- Tiered Architecture: Edge clusters for data collection, core clusters for processing, and specialized clusters for analytics
Kafka's MirrorMaker2 enables data replication between these clusters, supporting both active-passive and active-active configurations.
2. Schema Management
As data volumes grow, schema management becomes critical for ensuring data compatibility and evolution:
- Schema Registry: Central repository for Avro, JSON Schema, or Protobuf schemas
- Compatibility Rules: Forward, backward, or full compatibility enforcement
- Schema Evolution: Safe addition of optional fields and reasonable defaults
# Example Avro schema with evolution-friendly design
{
"type": "record",
"namespace": "com.example",
"name": "CustomerEvent",
"version": 1,
"fields": [
{"name": "customer_id", "type": "string"},
{"name": "event_type", "type": "string"},
{"name": "timestamp", "type": "long", "logicalType": "timestamp-millis"},
{"name": "properties", "type": {"type": "map", "values": "string"}, "default": {}}
]
}
3. Event Sourcing and CQRS
Kafka enables powerful architectural patterns that leverage its event log as the system of record:
- Event Sourcing: Storing state changes as an immutable sequence of events
- Command Query Responsibility Segregation (CQRS): Separating write and read models
- Materialized Views: Deriving specialized read models from event streams
- Event Replay: Reconstructing state by replaying events from any point
These patterns are particularly powerful for complex domains with audit requirements or systems that benefit from temporal queries (e.g., "what was the state at time T?").
4. Stream Processing Topologies
Real-time analytics and data transformations are implemented through stream processing applications:
- Kafka Streams: Lightweight client library for stream processing within applications
- ksqlDB: SQL interface for stream processing on Kafka
- Apache Flink: Distributed processing engine with advanced windowing and stateful operations
- Exactly-once Semantics: Processing guarantees for data transformation accuracy
// Example Kafka Streams topology for order enrichment
StreamsBuilder builder = new StreamsBuilder();
// Input topics
KStream orders = builder.stream("orders");
KTable customers = builder.table("customers");
// Join orders with customer data
KStream enrichedOrders = orders
.selectKey((k, v) -> v.getCustomerId())
.join(
customers,
(order, customer) -> new EnrichedOrder(order, customer)
);
// Output to enriched orders topic
enrichedOrders.to("enriched-orders");
Performance Optimization and Tuning
Optimizing Kafka for high-throughput, low-latency environments requires attention to multiple layers of the stack:
Hardware Considerations
Kafka's performance is heavily influenced by the underlying infrastructure:
- Disk I/O: SSDs or high-performance HDDs with separate volumes for logs and OS
- Network: 10+ Gbps networking to handle high-throughput replication
- Memory: Sufficient RAM for page cache to optimize read operations
- CPU: Multiple cores for parallel request processing and compression
Broker Configuration
Key broker settings that impact performance include:
- num.replica.fetchers: Threads used for replication (scale with broker count)
- num.network.threads / num.io.threads: Scale with client connections
- log.retention.bytes / log.retention.hours: Balance retention with disk usage
- log.segment.bytes: Impact on file handling and deletion efficiency
Producer Optimization
High-throughput producers benefit from:
- batch.size: Larger batches improve throughput at the cost of latency
- linger.ms: Waiting time to accumulate more records in a batch
- compression.type: snappy, lz4, or zstd depending on CPU/network tradeoffs
- acks: Durability vs. throughput tradeoff (0, 1, or all)
Consumer Optimization
Efficient consumption strategies include:
- fetch.min.bytes / fetch.max.wait.ms: Balance latency and efficient fetching
- max.poll.records: Control batch size for processing
- enable.auto.commit: Tradeoff between convenience and control
- Parallel Processing: Using multiple threads to process batches while maintaining ordering
Monitoring and Observability
Comprehensive monitoring is essential for production Kafka clusters:
Key Metrics to Track
- Broker Metrics: CPU, memory, disk usage, network throughput
- Under-replicated Partitions: Indicates replication issues
- Request Rate and Latencies: Produce/fetch performance
- Consumer Lag: Difference between latest message and consumer position
- Partition Count: Total partitions per broker (capacity planning)
Monitoring Tools
- Prometheus/Grafana: Open-source metrics collection and visualization
- Confluent Control Center: Commercial monitoring solution
- Kafka Manager/CMAK: Cluster management and monitoring UI
- LinkedIn's Burrow: Advanced consumer lag detection
Security and Compliance
Enterprise Kafka deployments require robust security measures:
- Authentication: SASL mechanisms (PLAIN, SCRAM, Kerberos, OAuth)
- Authorization: ACL-based permission control for topics and consumer groups
- Encryption: TLS for in-flight encryption, encryption at rest for sensitive data
- Audit Logging: Tracking access and administrative operations
- Data Governance: Subject mapping, classification, and lineage tracking
Common Operational Challenges and Solutions
Based on real-world experience, these are the most frequent challenges teams encounter:
Challenge | Symptoms | Solution |
---|---|---|
Consumer Lag | Delayed processing, growing offset difference | Scale consumers, optimize processing, increase partition count |
Broker Failures | Under-replicated partitions, offline partitions | Adequate replication factor, rack awareness, automated recovery |
Unbalanced Clusters | Uneven load distribution, some brokers overloaded | Kafka Cruise Control, partition reassignment, strategic topic design |
Topic Sprawl | Excessive topics/partitions, metadata overhead | Topic naming conventions, lifecycle policies, consolidation |
Scaling Kafka for the Enterprise
As Kafka deployments mature, these strategies help scale the platform effectively:
Organizational Scaling
- Platform Team Model: Centralized expertise with self-service capabilities
- Topic Ownership: Clear responsibility for schemas and retention policies
- SLAs and Capacity Planning: Formal agreements for throughput and availability
- Change Management: Controlled processes for configuration changes
Technical Scaling
- Tiered Storage: Separating hot and cold data across storage tiers
- Multi-Datacenter Replication: Active-active or active-passive setups
- Kafka Connect Ecosystem: Standardized integration with external systems
- Topic Compaction: Key-based retention for state-oriented topics
Use Cases and Design Patterns
Kafka excels in various scenarios, each with specific design considerations:
Log Aggregation
Centralizing logs from distributed systems:
- High partition count for parallel processing
- Time-based retention policies
- Compression for storage efficiency
Metrics Collection
Real-time monitoring data:
- Topic partitioning by metric source
- Sampling and aggregation for high-frequency metrics
- Retention aligned with monitoring needs
Event-Driven Microservices
Service communication through events:
- Event schema design with forward compatibility
- Idempotent consumers for resilience
- Dead letter queues for error handling
Real-time Analytics
Processing data streams for insights:
- Stateful stream processing for aggregations
- Windowing strategies for time-based analysis
- Materialized views for query optimization
The Future of Kafka and Event Streaming
Kafka continues to evolve with several emerging trends:
- KRaft Mode: ZooKeeper-free Kafka for simplified architecture
- Tiered Storage: Decoupling storage from compute for cost-effective scaling
- Serverless Kafka: Managed offerings with consumption-based pricing
- Stream Governance: Advanced data lineage, quality, and catalog integration
- Real-time ML/AI: Stream processing for machine learning pipelines
Conclusion
Apache Kafka has transformed how organizations think about and implement data flows. By providing a durable, scalable foundation for event-driven architectures, Kafka enables real-time data processing that was previously impractical at enterprise scale.
However, success with Kafka requires thoughtful architecture, operational discipline, and continuous optimization. The patterns and practices outlined in this article reflect years of hands-on experience building mission-critical systems with Kafka at their core.
As real-time data becomes increasingly central to competitive advantage, mastering platforms like Kafka will remain an essential skill for data engineers, architects, and DevOps professionals navigating the evolving data landscape.
Related Articles
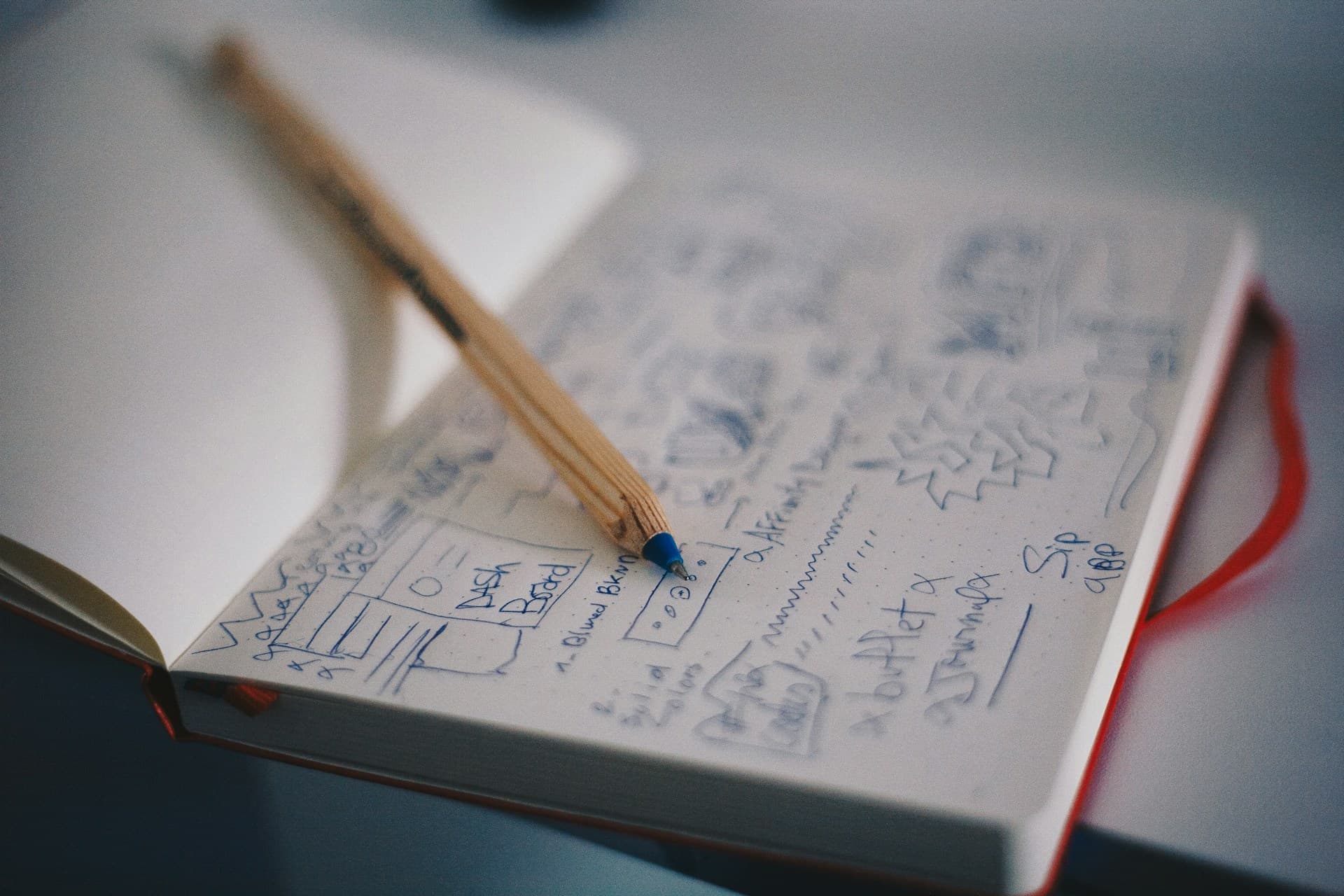
Essential Microservices Design Patterns for Enterprise Architectures
Explore proven microservices design patterns that solve complex distributed systems challenges. From service discovery and resilient communication to data consistency and scalable deployment strategies—learn how seasoned architects implement production-grade microservices ecosystems.
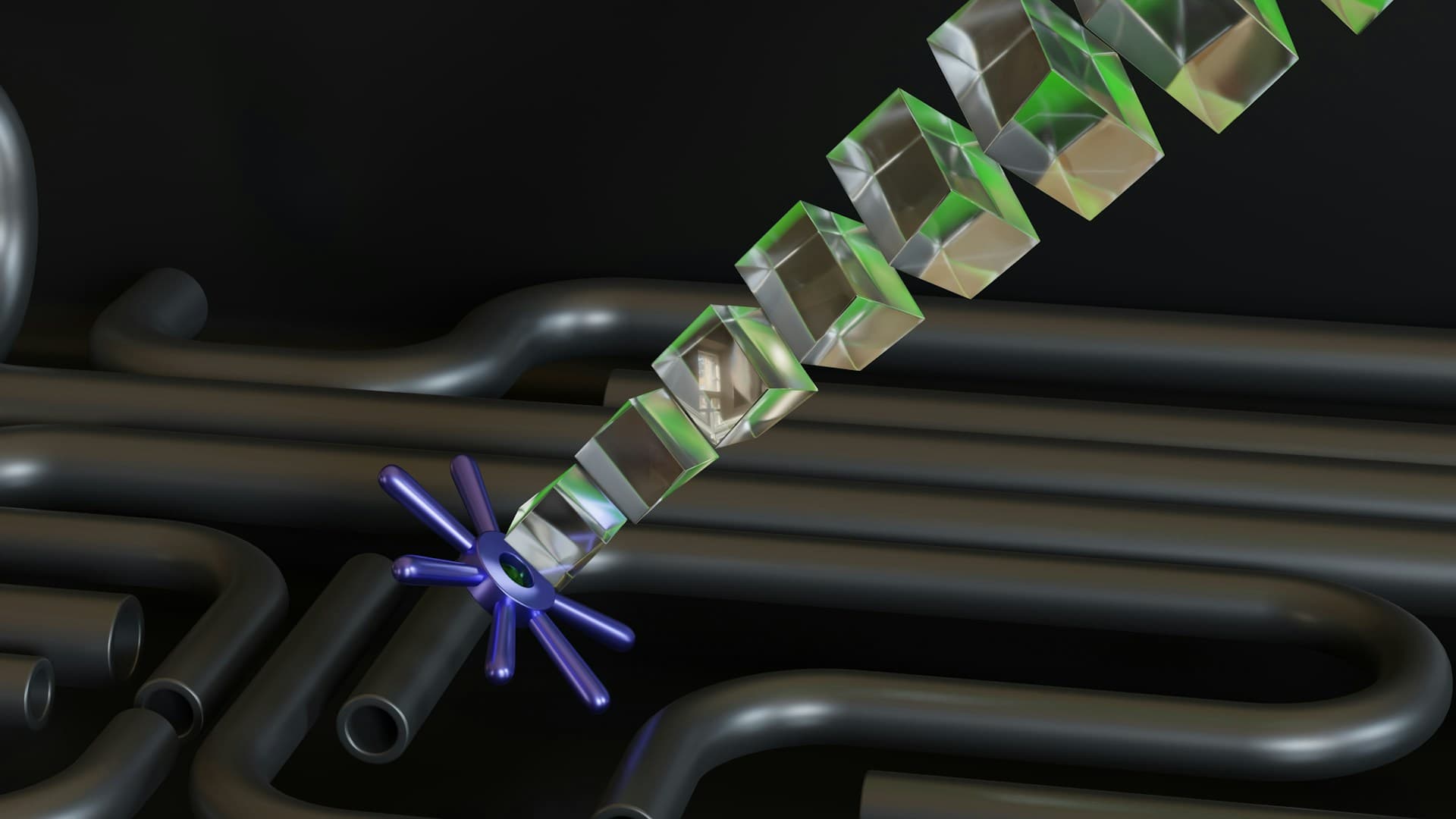
Kubernetes for Beginners: A Comprehensive Guide
Kubernetes has become the industry standard for container orchestration. This guide will help you understand the basics and get started with your first cluster.
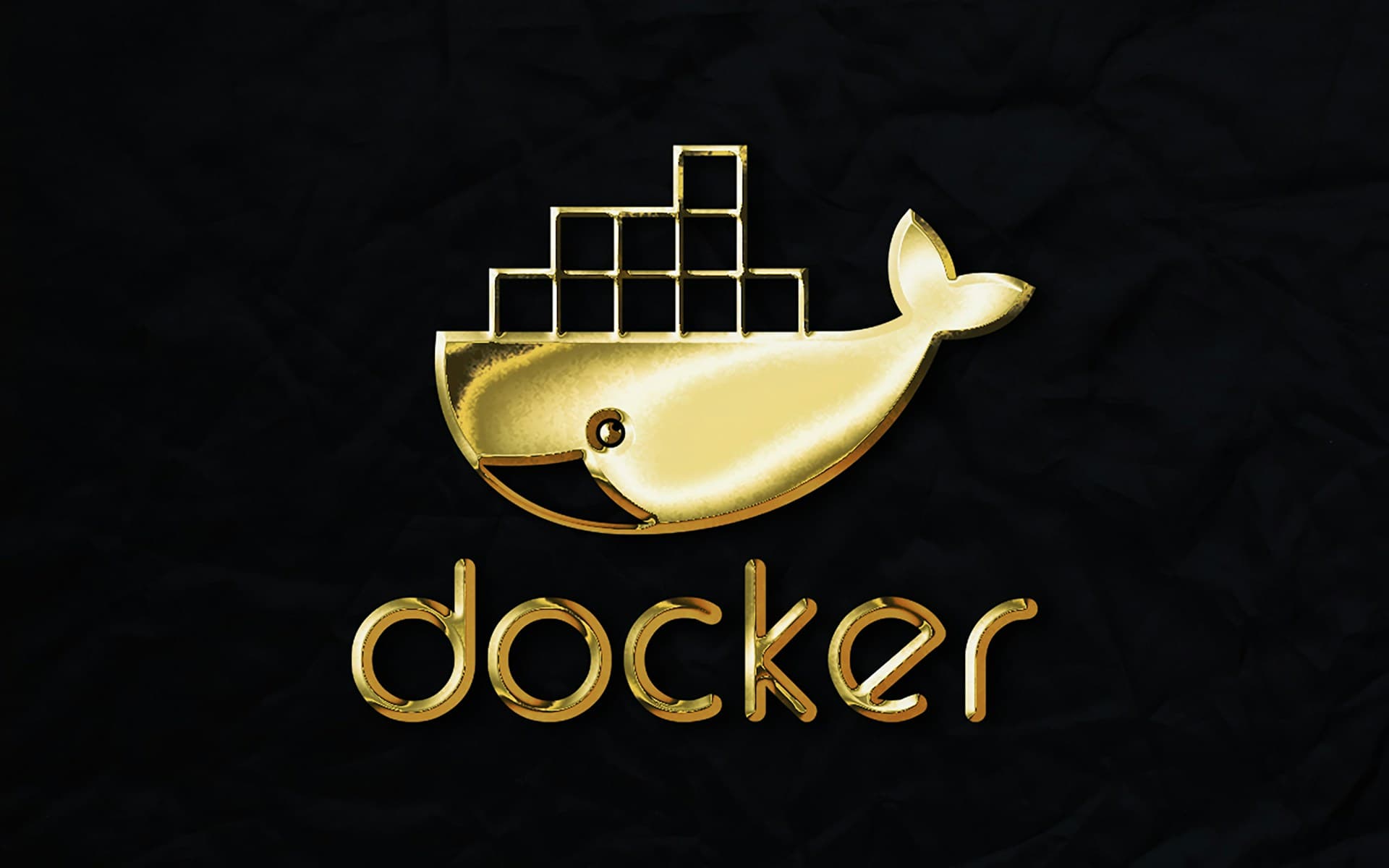
Enterprise-Grade Docker: Production Hardening and Optimization Techniques
Master production-ready containerization with battle-tested Docker best practices. Learn advanced security hardening, performance optimization, resource governance, and orchestration strategies derived from real-world enterprise deployments.