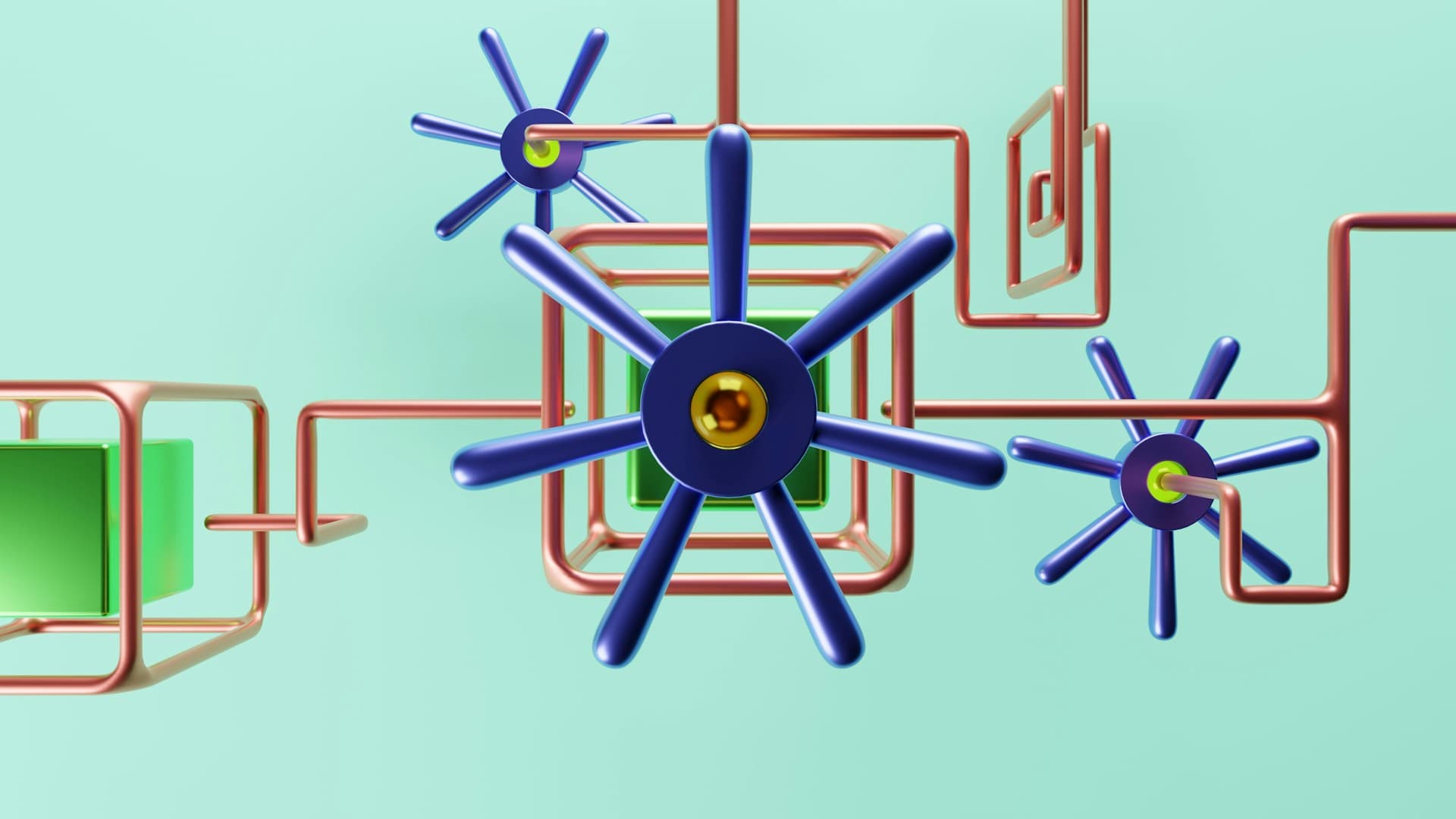
Continuous Integration and Continuous Delivery (CI/CD) are essential practices for modern software development. Learn the best practices for implementing effective CI/CD pipelines.
After implementing CI/CD pipelines in enterprise environments for over a decade, I've witnessed firsthand how Continuous Integration (CI) and Continuous Delivery (CD) transform software delivery. These methodologies have evolved from emerging practices to industry standards that enable development teams to ship high-quality code changes with greater frequency, reliability, and confidence.
The Business Value of CI/CD Adoption
Organizations that effectively implement CI/CD pipelines gain significant competitive advantages through:
- Accelerated time-to-market: Reduce deployment cycles from weeks to hours or minutes
- Enhanced code quality: Catch issues early through automated testing and code analysis
- Reduced operational overhead: Minimize manual intervention and human error in deployment processes
- Increased developer productivity: Allow engineers to focus on delivering features rather than managing deployments
- Improved release reliability: Consistently deploy working software through repeatable, tested processes
- Better team collaboration: Break down silos between development, operations, and QA teams
Elements of a Mature CI/CD Pipeline
A production-grade CI/CD pipeline typically consists of these interconnected stages:
Pipeline Stage | Key Components | Automation Goals |
---|---|---|
Source | Version control, code reviews, branch policies | Ensure code quality at the source |
Build | Compilation, linting, static analysis | Create consistent, reproducible artifacts |
Test | Unit, integration, performance testing | Verify functionality and performance |
Security | SAST, DAST, dependency scanning | Identify vulnerabilities early |
Deploy | Environment provisioning, deployment automation | Provide consistent, reliable releases |
Operate | Monitoring, alerting, observability | Ensure production stability |
CI/CD Pipeline Best Practices
Through years of implementation across various industries and technology stacks, I've identified these essential best practices for creating robust CI/CD pipelines:
1. Optimize Pipeline Performance
Pipeline execution time directly impacts developer productivity and feedback cycles. In high-velocity teams, aim for pipelines that complete in under 10 minutes for most changes:
- Parallel execution: Run independent tests and tasks concurrently to reduce total execution time
- Incremental builds: Only rebuild components affected by recent changes
- Strategic caching: Cache dependencies, build artifacts, and test resources between pipeline runs
- Test segmentation: Create fast feedback loops with quick tests, followed by more comprehensive suites
- Distributed testing: Split test suites across multiple agents or containers
# Example: Jenkins pipeline with parallelization
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean compile'
}
}
stage('Test') {
parallel {
stage('Unit Tests') {
steps {
sh 'mvn test'
}
}
stage('Integration Tests') {
steps {
sh 'mvn verify -DskipUnitTests'
}
}
stage('Security Scan') {
steps {
sh 'mvn dependency-check:check'
}
}
}
}
}
}
2. Ensure Pipeline Reliability
A pipeline is only valuable if teams trust its results. Unreliable pipelines lead to workarounds and process breakdowns:
- Hermetic builds: Create isolated, deterministic build environments using containers
- Test environment parity: Minimize differences between testing and production environments
- Flaky test management: Identify, quarantine, and fix unstable tests
- Graceful failure handling: Implement retry mechanisms for transient failures (network issues, resource contention)
- Pipeline analytics: Track pipeline health metrics and continuously improve reliability
3. Implement Comprehensive Testing Strategies
Quality validation is the cornerstone of CI/CD confidence. Structure your testing pyramid effectively:
- Unit tests: Fast, focused tests that validate individual components in isolation (70-80% of test suite)
- Integration tests: Verify interactions between components (15-20% of test suite)
- End-to-end tests: Validate complete user journeys and system behavior (5-10% of test suite)
- Contract tests: Ensure API compatibility between services
- Performance tests: Verify system performance under load
- Chaos testing: Validate system resilience during failures
4. Embrace Infrastructure as Code (IaC)
Treat infrastructure with the same discipline as application code to ensure consistency and reproducibility:
- Environment templates: Define all infrastructure components (compute, network, storage) as code
- Configuration management: Manage application settings through version-controlled configuration
- Environment provisioning: Automate creation and destruction of environments
- Immutable infrastructure: Replace rather than modify infrastructure components
- Multi-environment promotion: Use the same deployment process across development, staging, and production
# Example: Terraform configuration for application infrastructure
resource "aws_ecs_service" "app_service" {
name = "application"
cluster = aws_ecs_cluster.main.id
task_definition = aws_ecs_task_definition.app.arn
desired_count = 3
launch_type = "FARGATE"
deployment_controller {
type = "CODE_DEPLOY"
}
network_configuration {
security_groups = [aws_security_group.app_sg.id]
subnets = aws_subnet.private[*].id
assign_public_ip = false
}
load_balancer {
target_group_arn = aws_lb_target_group.blue.arn
container_name = "application"
container_port = 8080
}
lifecycle {
ignore_changes = [task_definition, load_balancer]
}
}
5. Integrate Security Throughout the Pipeline
Security must be a continuous concern, not a final gate. Implement these DevSecOps practices:
- Dependency scanning: Automatically identify vulnerable dependencies
- Static Application Security Testing (SAST): Analyze source code for security vulnerabilities
- Dynamic Application Security Testing (DAST): Test running applications for exploitable vulnerabilities
- Container scanning: Check container images for vulnerabilities and misconfigurations
- Infrastructure scanning: Validate infrastructure definitions against security best practices
- Secrets management: Securely handle credentials and sensitive configuration
6. Implement Progressive Delivery
Reduce deployment risk through controlled rollout strategies:
- Feature flags: Decouple deployment from feature release
- Blue/green deployments: Run two identical environments and switch traffic between them
- Canary releases: Gradually route traffic to new versions
- A/B testing: Compare different implementations with metrics-based evaluation
- Automated rollbacks: Rapidly revert to previous versions when issues are detected
7. Establish Meaningful Metrics
Measure your CI/CD performance to identify bottlenecks and drive improvements:
- Lead time: Time from code commit to production deployment
- Deployment frequency: How often you deploy to production
- Change failure rate: Percentage of deployments causing production issues
- Mean time to recovery: Average time to restore service after failures
- Pipeline throughput: Number of changes processed per day
- Quality metrics: Code coverage, technical debt, security findings
Implementing CI/CD at Scale
For enterprise organizations with complex environments, consider these additional strategies:
Pipeline as Code
Define CI/CD pipelines as version-controlled code to ensure consistency, enable reviews, and facilitate reuse:
# Example: GitLab CI pipeline configuration
stages:
- build
- test
- security
- deploy-staging
- integration
- deploy-production
variables:
DOCKER_DRIVER: overlay2
DOCKER_TLS_CERTDIR: ""
build:
stage: build
image: docker:20.10.16
services:
- docker:20.10.16-dind
script:
- docker build -t $CI_REGISTRY_IMAGE:$CI_COMMIT_SHA .
- docker push $CI_REGISTRY_IMAGE:$CI_COMMIT_SHA
cache:
key: "$CI_COMMIT_REF_SLUG"
paths:
- .npm/
- node_modules/
Monorepo CI/CD Strategies
For monorepo architectures, implement these optimizations:
- Selective builds based on changed files
- Dependency graphs to determine affected services
- Distributed caching for build artifacts
- Coordinated versioning and releases
Self-Service Developer Platforms
Create internal platforms that enable development teams to implement CI/CD without deep DevOps expertise:
- Template-based pipeline creation
- Standardized deployment processes
- Automated compliance guardrails
- Centralized observability and monitoring
Common CI/CD Pitfalls and Solutions
Even experienced teams encounter these common challenges:
Challenge | Impact | Solution |
---|---|---|
Slow pipelines | Reduced developer productivity, delayed feedback | Implement parallelization, caching, and test optimization |
Flaky tests | Lost trust in pipeline, wasted debugging time | Quarantine flaky tests, improve test isolation |
Environment inconsistency | "Works on my machine" issues, production bugs | Use containers, IaC, and environment parity |
Pipeline complexity | Difficult maintenance, tribal knowledge | Modularize pipelines, create reusable components |
CI/CD Tools and Ecosystem
The CI/CD landscape offers various options to fit different requirements:
Self-Hosted Options
- Jenkins: Highly customizable with extensive plugin ecosystem
- GitLab CI: Integrated with GitLab SCM for streamlined workflows
- TeamCity: Enterprise-grade CI/CD with advanced caching and build grid
- Bamboo: Tight integration with Atlassian tools
Cloud-Based Services
- GitHub Actions: Native CI/CD for GitHub repositories
- CircleCI: Developer-friendly CI/CD with powerful caching
- AWS CodePipeline: Integrated with AWS services ecosystem
- Azure DevOps Pipelines: Enterprise-ready with strong Windows support
- Google Cloud Build: Serverless CI/CD integrated with GCP
Specialized CI/CD Tools
- Spinnaker: Multi-cloud continuous delivery platform
- ArgoCD: Kubernetes-native continuous delivery
- Tekton: Cloud-native CI/CD building blocks
- Harness: AI-powered continuous delivery platform
The Future of CI/CD
The CI/CD space continues to evolve with these emerging trends:
- GitOps: Git-centric approach to infrastructure and deployment automation
- AI/ML in CI/CD: Intelligent test selection, anomaly detection, and predictive analytics
- Platform engineering: Internal developer platforms abstracting CI/CD complexity
- Infrastructure from code: Generating infrastructure based on application requirements
- Shift-right testing: Runtime validation, chaos engineering, and production testing
Conclusion
A well-designed CI/CD pipeline is a strategic asset that enables your development teams to deliver value faster while maintaining high quality and security standards. By following these best practices and continuously improving your pipeline, you can achieve the agility needed in today's competitive software landscape.
Remember that CI/CD implementation is a journey, not a destination. Start with foundational capabilities, measure your results, and iteratively enhance your processes based on team feedback and evolving business needs.
Related Articles
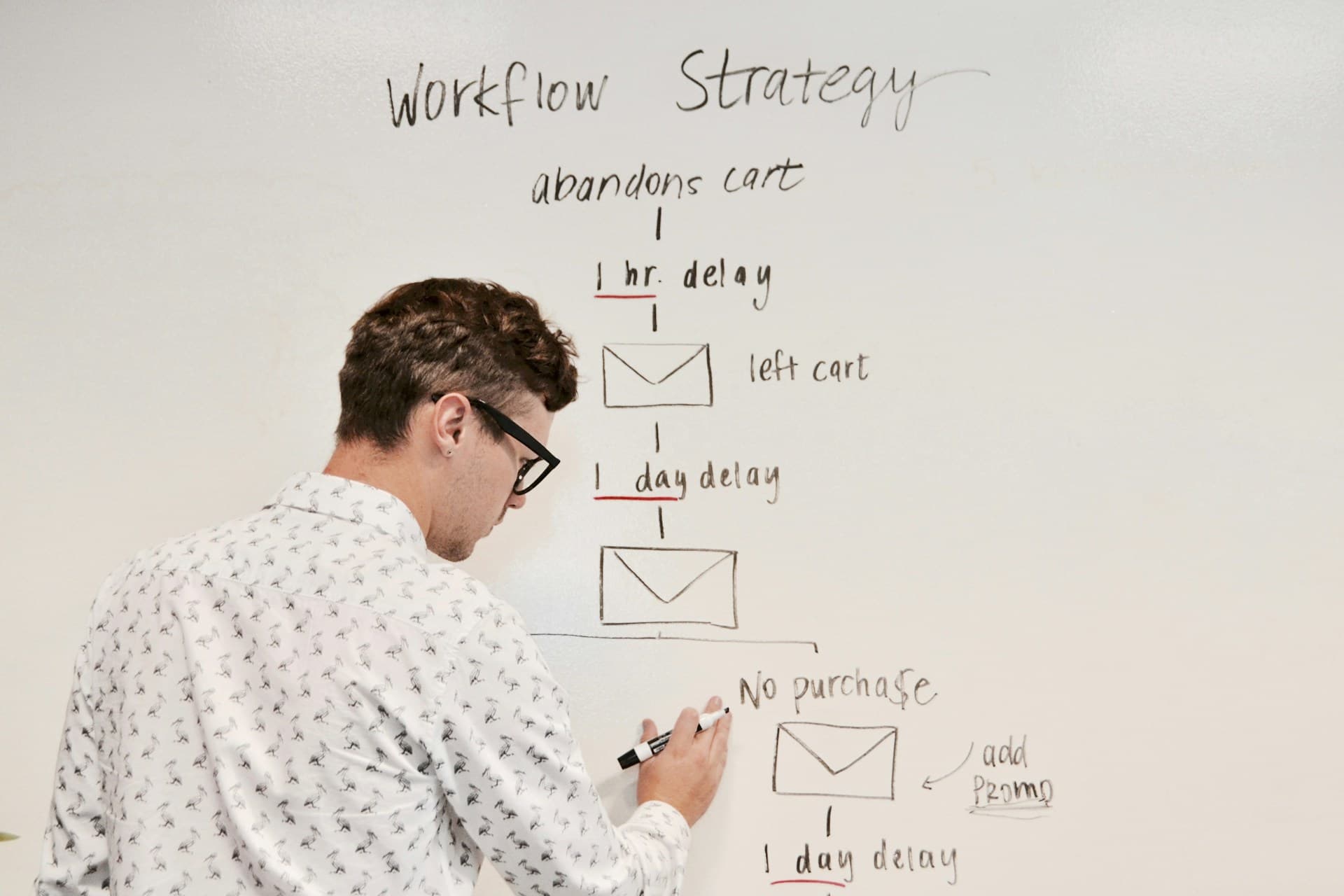
Implementing GitOps: Declarative Infrastructure and Continuous Delivery with ArgoCD
Discover how GitOps revolutionizes Kubernetes deployments by leveraging Git as the single source of truth. Learn how to implement production-grade GitOps workflows with ArgoCD to achieve automated, auditable, and reliable continuous delivery pipelines.
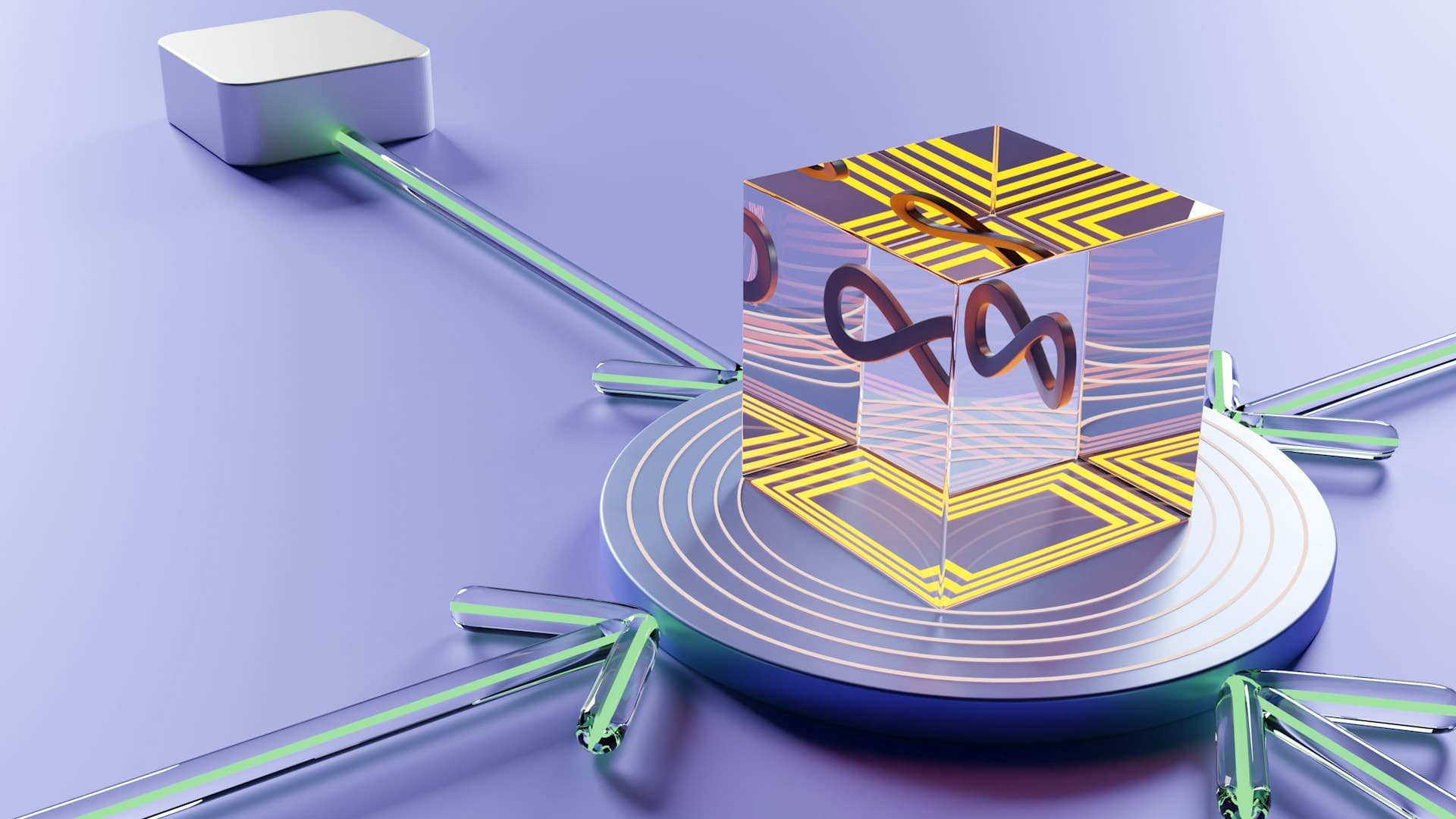
Introduction to DevOps: Bridging Development and Operations
DevOps is a set of practices that combines software development (Dev) and IT operations (Ops). It aims to shorten the systems development life cycle and provide continuous delivery with high software quality.
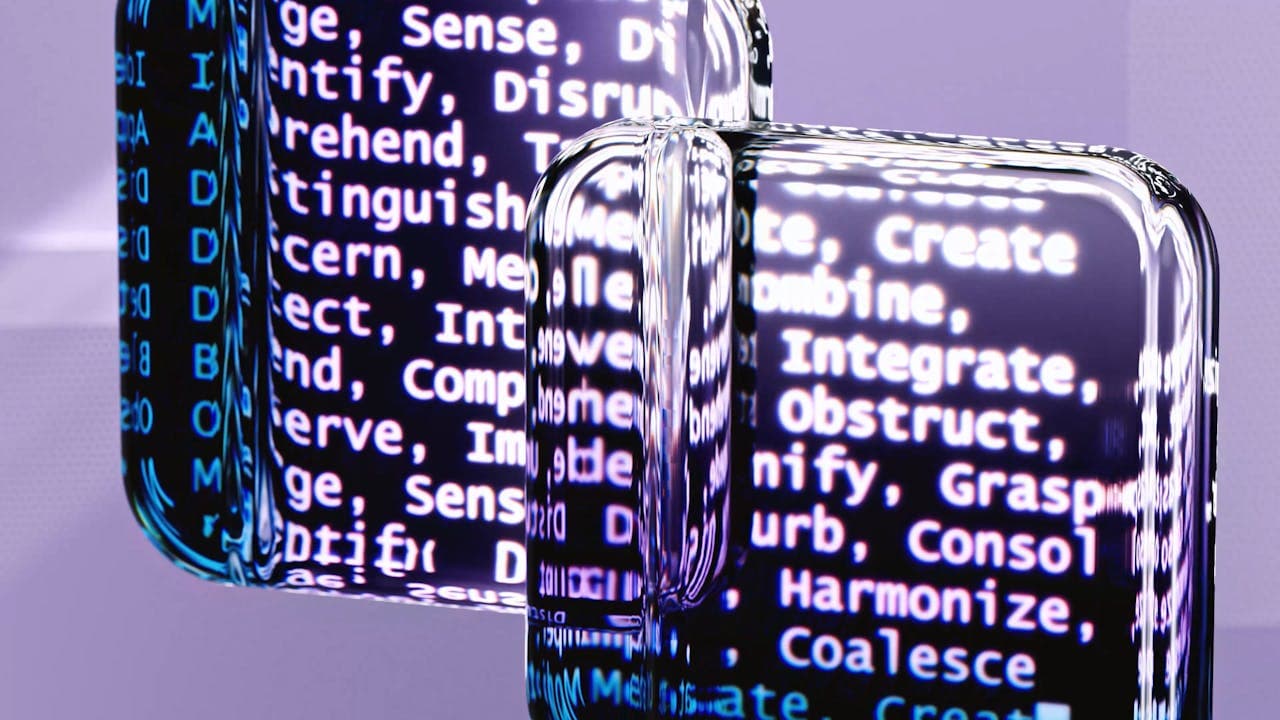
Enterprise Infrastructure as Code: Architecting Cloud-Native Platforms with Terraform
Discover how to implement infrastructure as code (IaC) at scale with Terraform. Learn battle-tested patterns for managing complex, multi-cloud environments, state management strategies, and CI/CD pipeline integration from a senior platform engineering perspective.