Enterprise-Grade Docker: Production Hardening and Optimization Techniques
February 25, 2025
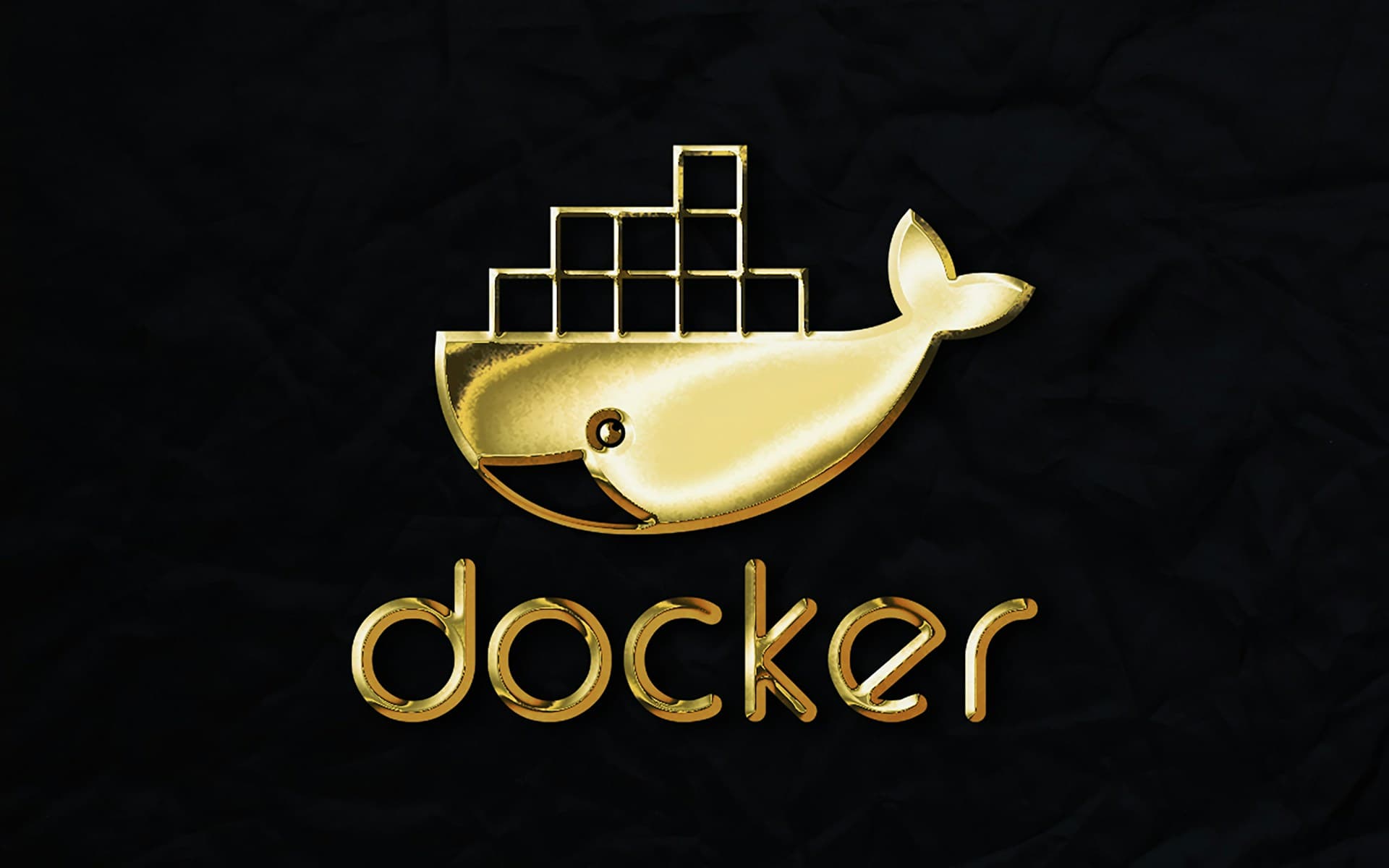
Master production-ready containerization with battle-tested Docker best practices. Learn advanced security hardening, performance optimization, resource governance, and orchestration strategies derived from real-world enterprise deployments.
After implementing Docker-based solutions in production environments across financial services, healthcare, and e-commerce sectors for the past decade, I've observed a consistent pattern: the gap between basic containerization and production-ready deployments remains substantial. While spinning up containers for development is straightforward, building secure, scalable, and operationally mature containerized systems requires deeper engineering discipline. This guide distills critical lessons from architecting and operating container platforms handling millions of daily transactions in regulated environments.
Image Security and Supply Chain Integrity
Base Image Selection and Hardening
Your security posture begins with base image selection. Throughout my work with security-sensitive organizations, I've established these foundational practices:
- Minimal Base Images - Prefer Alpine or distroless images to reduce attack surface. Each additional package or library increases vulnerability exposure and image size.
- Verified Sources - Pull base images only from trusted registries with signature verification enabled. My teams implement Notary or Cosign to verify image authenticity.
- Regular Rebasing - Automate periodic rebuilds (typically weekly) of all images to incorporate security patches from base images.
- Version Pinning - Never use "latest" tags in production. Pin to specific digests (SHA256) rather than tags to ensure image immutability.
A security-hardened Dockerfile for a Node.js application follows this pattern:
# Multi-stage build for minimal production image
FROM node:18.19-alpine3.18 AS build
# Set working directory
WORKDIR /app
# Copy dependency definitions and install with exact versions
COPY package*.json ./
RUN npm ci --production
# Copy application code
COPY . .
# Build application
RUN npm run build
# Create production image
FROM node:18.19-alpine3.18 AS production
# Set non-root user and working directory
WORKDIR /app
RUN addgroup -g 1001 appuser && adduser -u 1001 -G appuser -s /bin/sh -D appuser && chown -R appuser:appuser /app
# Copy from build stage
COPY --from=build --chown=appuser:appuser /app/node_modules ./node_modules
COPY --from=build --chown=appuser:appuser /app/dist ./dist
COPY --from=build --chown=appuser:appuser /app/package.json ./
# Security hardening
RUN apk update && apk upgrade && # Remove unnecessary tools
apk del curl tar rpm && # Clean package manager cache
rm -rf /var/cache/apk/* && # Reduce permissions
chmod -R 550 /app && find /app -type f -exec chmod 440 {} ;
# Configure runtime
USER appuser
ENV NODE_ENV=production
ENV PORT=8080
EXPOSE 8080
# Define healthcheck
HEALTHCHECK --interval=30s --timeout=5s --retries=3 CMD wget -q -O - http://localhost:8080/health || exit 1
# Run application with reduced privileges
CMD ["node", "dist/server.js"]
Image Scanning and Vulnerability Management
Vulnerability management is an ongoing process, not a one-time check. Based on implementing security pipelines for regulated industries, I recommend:
- Multi-Layer Scanning - Scan base images, application dependencies, and final images using tools like Trivy, Clair, or Snyk as part of your CI pipeline.
- Risk-Based Remediation - Focus on fixing vulnerabilities with known exploits and those affecting components directly accessible externally first.
- Build-Time Blocking - Configure CI pipelines to fail builds with critical vulnerabilities in production images, with bypass requiring explicit security team approval.
- Runtime Detection - Implement drift detection between built and running images using admission controllers in Kubernetes (e.g., OPA Gatekeeper or Kyverno).
Our most mature clients implement automated vulnerability management with this workflow:
Stage | Actions | Automation |
---|---|---|
Pre-Build | Base image scanning, dependency analysis | Scheduled weekly scans, PR-triggered scans |
Build-Time | SBOM generation, policy compliance checks | CI pipeline integration, artifact signing |
Registry | Image vulnerability scanning, policy enforcement | Registry scanning hooks, promotion policies |
Runtime | Container security monitoring, drift detection | Admission controllers, runtime security agents |
Performance Optimization Strategies
Image Size Reduction
Smaller images offer numerous benefits: faster deployments, reduced attack surface, and better resource utilization. Having optimized hundreds of container images for production, I recommend these techniques:
- Multi-stage Builds - Separate build environments from runtime environments to eliminate build tools and intermediate artifacts from final images.
- Layer Optimization - Combine related RUN commands to reduce layer count while preserving cache efficiency for frequently changing components.
- .dockerignore - Maintain comprehensive .dockerignore files to prevent unnecessary files (logs, tests, documentation) from bloating your image.
- Dependency Pruning - Remove development dependencies after building and implement tools like node-prune for JavaScript applications.
With these techniques, we typically achieve 50-80% reduction in image size compared to naive approaches. For example, a React application image was reduced from 1.2GB to 87MB using these methods.
Resource Governance and Constraints
Proper resource allocation is essential for stable production environments. Based on my experience managing large-scale container clusters, I recommend:
- Memory Limits - Always set explicit memory limits matching application requirements to prevent resource contention and OOM kills.
- CPU Constraints - Set CPU requests based on baseline needs and limits based on peak usage patterns plus headroom.
- Init Sizing Analysis - Analyze application startup patterns to correctly size for initialization, which often requires more resources than steady-state operation.
- Tagging Strategy - Implement detailed resource tagging to enable accurate cost allocation and utilization analysis.
For Kubernetes environments, my standard resource configuration pattern includes explicit resource requests and limits, properly configured health probes, graceful shutdown handling, and security context settings.
Container Orchestration Best Practices
Service Discovery and Networking
Production containerized applications require robust networking. Having migrated monolithic applications to microservices architectures, I recommend:
- Internal Service Mesh - Implement a service mesh (Istio, Linkerd, or Consul) for applications with complex service-to-service communication to handle routing, encryption, and observability.
- Network Policies - Define explicit network policies that restrict container communication to only what's necessary, following zero-trust principles.
- DNS Caching - Configure appropriate DNS caching for containers to prevent resolution storms during scaling events.
- Connection Pooling - Implement connection pooling for database and service connections to reduce connection establishment overhead.
Persistent Data Management
Containers are ephemeral, but data often needs to persist. Based on implementing stateful services in containerized environments, these practices ensure data durability:
- Volume Abstraction - Use storage abstraction layers (Kubernetes PVCs, Docker named volumes) instead of direct host mounts to enable platform portability.
- Data Lifecycle Alignment - Align volume lifecycle with data sensitivity – ephemeral for scratch data, persistent for business records, with appropriate backup strategies.
- Database Containerization - Follow specialized best practices for containerized databases, including dedicated nodes, anti-affinity rules, and proper volume sizing.
- Backup Automation - Implement automated backup processes with validation for all persistent container volumes.
Observability and Monitoring
Logging Strategies
Effective logging is critical for troubleshooting containerized applications. From implementing observability platforms for large-scale deployments, I recommend:
- Stdout/Stderr Output - Direct all application logs to stdout/stderr to leverage container runtime logging drivers instead of file-based logging.
- Structured Logging - Implement structured JSON logging with consistent fields across all services for easier querying and analysis.
- Correlation IDs - Generate and propagate correlation IDs across service boundaries to trace requests through distributed systems.
- Log Aggregation - Implement centralized log aggregation (ELK stack, Loki, or cloud-native solutions) with retention policies aligned to compliance requirements.
Container Health Monitoring
Comprehensive monitoring enables proactive management of containerized applications. Having built observability platforms for production environments, I recommend:
- Multi-Dimensional Metrics - Collect container-level metrics (CPU, memory, I/O) alongside application-specific metrics for complete visibility.
- Prometheus Integration - Implement Prometheus exporters or compatible metrics endpoints in your applications for standardized metric collection.
- RED Method - Monitor Request rate, Error rate, and Duration for all services to provide consistent service health indicators.
- Custom Dashboards - Develop role-specific dashboards for operations, development, and business stakeholders with appropriate levels of detail.
CI/CD Pipeline Integration
Automated Build and Test
Integrating Docker into CI/CD pipelines enables consistent build, test, and deployment processes. Based on implementing GitOps workflows for large organizations, I recommend:
- Centralized Build Service - Implement build farms with controlled environments rather than relying on developer workstations for production images.
- Image Promotion Workflow - Establish explicit image promotion workflows across environments (dev, staging, production) with appropriate approvals.
- Reproducible Builds - Ensure deterministic builds by pinning all dependencies and eliminating time-based or random variations.
- Artifact Integrity - Implement container image signing and verification throughout the pipeline to maintain integrity.
Deployment Strategies
Containerization enables sophisticated deployment strategies that minimize risk. Having implemented deployment automation for mission-critical services, I recommend:
- Blue-Green Deployments - Maintain two identical environments with instant cutover capability to minimize deployment risk.
- Canary Releases - Roll out changes to a small subset of users or servers before full deployment to detect issues early.
- Feature Flags - Implement feature flagging to separate deployment from release, allowing controlled exposure of new features.
- Rollback Automation - Create automated rollback procedures triggered by health check failures or error rate thresholds.
Conclusion: Container Maturity Model
After helping dozens of organizations adopt container technologies, I've observed that containerization maturity evolves through distinct phases from initial exploration to standardization, optimization, and finally innovation. By systematically implementing the practices outlined in this guide, organizations can accelerate their container adoption journey, avoiding common pitfalls while maximizing the benefits of containerization.
Remember that container adoption is not merely a technology shift but requires corresponding evolution in processes, skills, and organizational culture to fully realize its potential. Organizations that approach containerization holistically, addressing both technical and organizational aspects, consistently achieve the greatest benefits in terms of deployment velocity, operational reliability, and developer productivity.
Related Articles
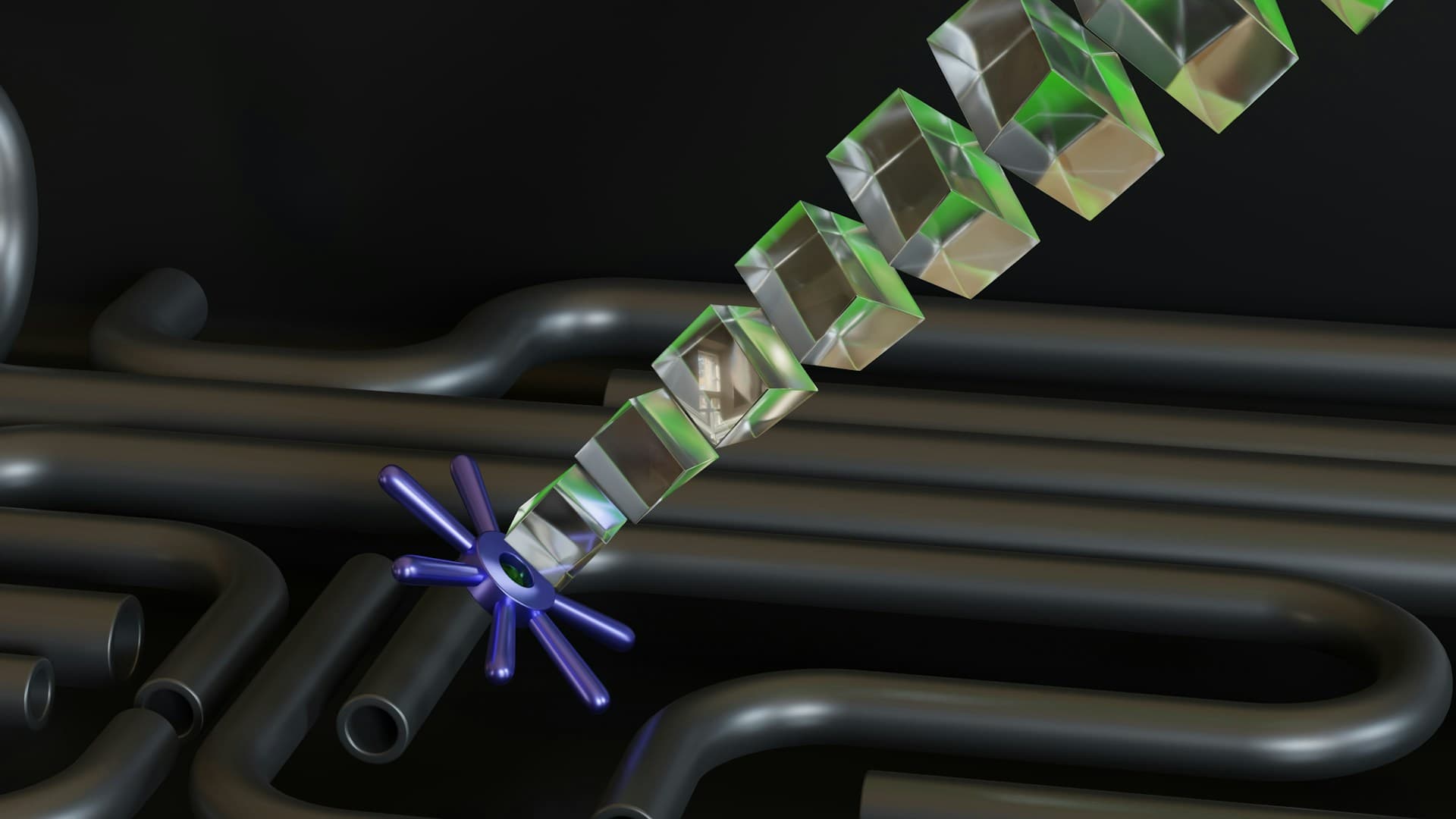
Kubernetes for Beginners: A Comprehensive Guide
Kubernetes has become the industry standard for container orchestration. This guide will help you understand the basics and get started with your first cluster.
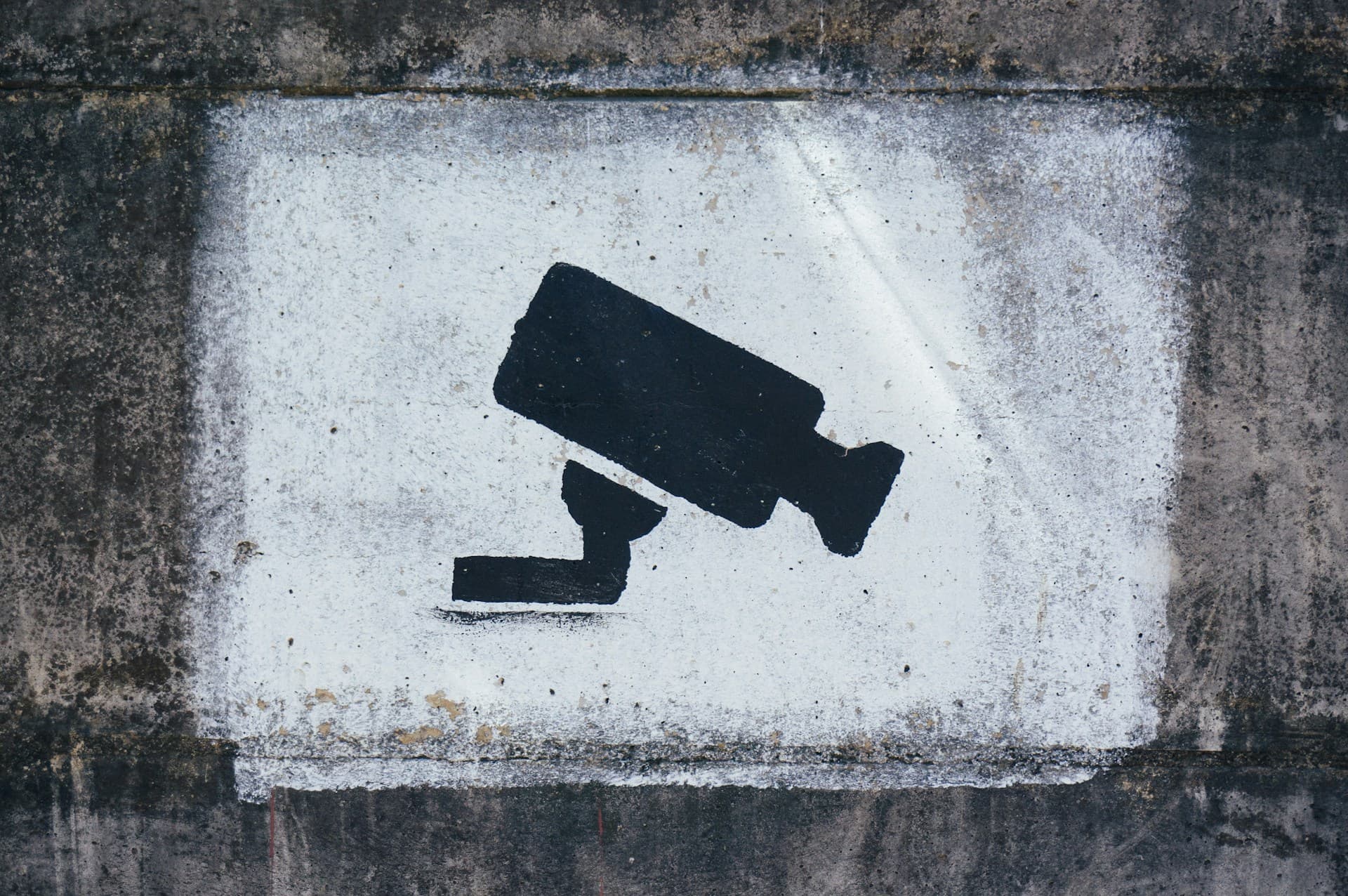
Enterprise Observability: Building Production-Grade Monitoring Systems with Prometheus and Grafana
Discover how to architect and implement scalable, high-availability observability platforms using Prometheus and Grafana. Learn proven strategies for metric collection, PromQL optimization, alerting hierarchies, and visualization best practices from an experienced SRE perspective.
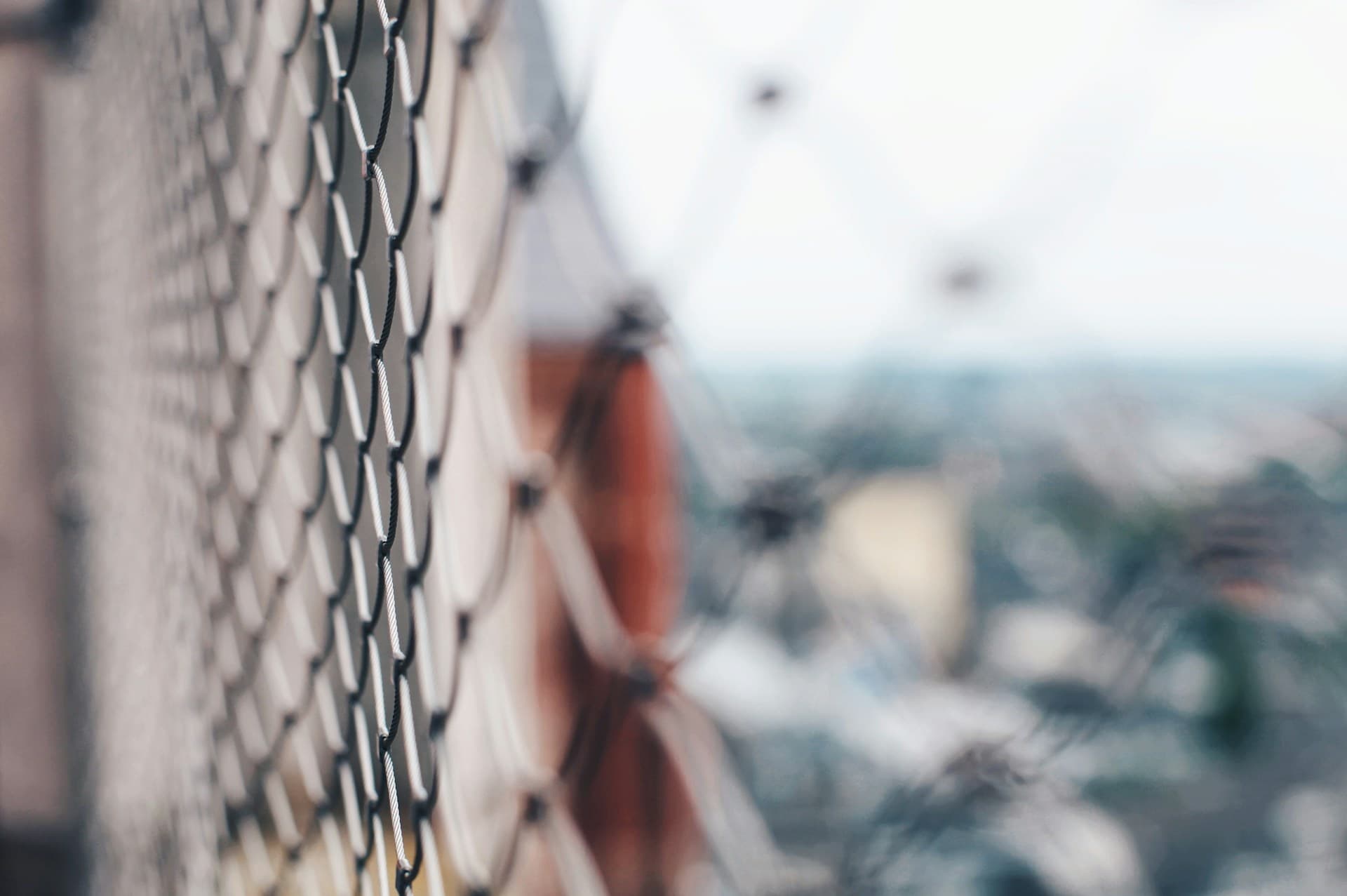
Service Mesh Architecture: The Critical Infrastructure Layer for Modern Microservices
Service mesh provides a dedicated infrastructure layer for managing service-to-service communication within microservices architectures. Learn how it enhances observability, security, and reliability in complex distributed systems.