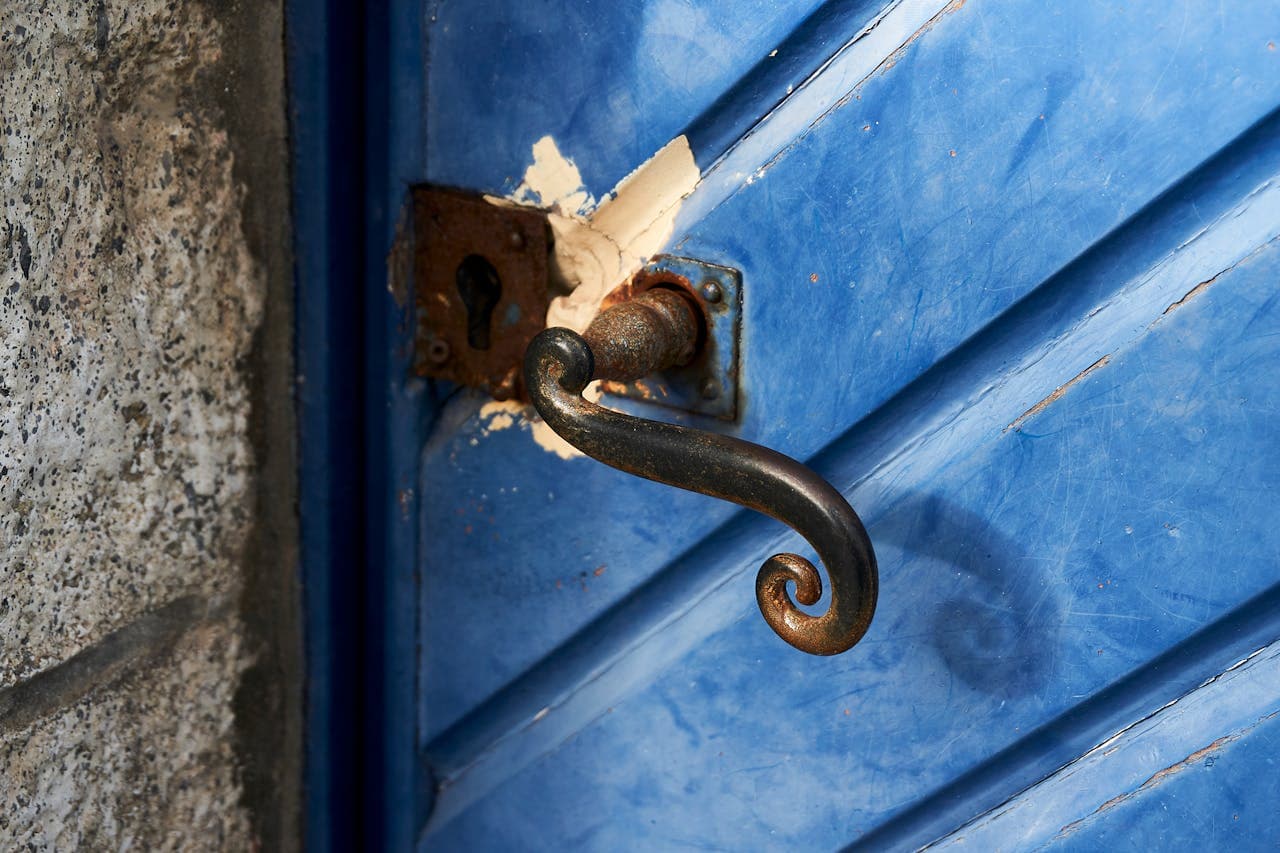
Discover how to seamlessly integrate security into your DevOps pipeline with DevSecOps methodologies. Learn battle-tested strategies, tools, and frameworks for automated vulnerability scanning, compliance monitoring, and secure infrastructure as code that won't slow down your delivery velocity.
After implementing security controls across hundreds of CI/CD pipelines over the past decade, I've witnessed the evolution of DevSecOps from a theoretical concept to an operational necessity. Organizations that fail to integrate security into their delivery pipelines face an existential risk in today's threat landscape—where vulnerable code can be exploited within minutes of deployment, and regulatory penalties for security breaches can cripple enterprises financially.
DevSecOps isn't just another industry buzzword; it's a fundamental shift in how technology organizations approach security—treating it as a shared responsibility baked into each phase of the software development lifecycle rather than a compliance gate at the end.
The Evolution of Security in Software Delivery
To appreciate how DevSecOps transforms security practices, we need to understand how application security has evolved:
Era | Security Approach | Limitations |
---|---|---|
Traditional (Pre-DevOps) | Security as a final gate before production | Late-stage discovery of vulnerabilities, resulting in costly rework and delays |
Early DevOps | Security as a separate concern, often bypassed for speed | Security debt accumulation, with velocity prioritized over safety |
DevSecOps | Security embedded throughout the SDLC | Requires cultural shift and tooling investments |
Next-Gen DevSecOps | AI-assisted security automation with proactive threat modeling | Still emerging, with technology rapidly evolving |
Core Principles of DevSecOps
Successful DevSecOps implementations are guided by these foundational principles:
1. Shift Security Left
Moving security earlier in the development process—ideally beginning at the design and requirements phase—prevents vulnerabilities from even entering your codebase. This approach is significantly more cost-effective than remediation after deployment:
- Threat modeling during design: Identify potential security risks before a single line of code is written
- Security requirements as user stories: Make security explicit in acceptance criteria
- Developer security training: Equip engineers with the knowledge to avoid common vulnerability patterns
- Pre-commit hooks: Scan code locally before it enters the shared repository
2. Automate Security Testing
Manual security reviews don't scale in fast-moving environments. Automation enables consistent, repeatable security testing that keeps pace with development:
- Static Application Security Testing (SAST): Analyze source code for security vulnerabilities
- Dynamic Application Security Testing (DAST): Test running applications to find runtime vulnerabilities
- Software Composition Analysis (SCA): Identify vulnerabilities in third-party components
- Infrastructure as Code (IaC) scanning: Validate infrastructure definitions against security best practices
- Container scanning: Check container images for known vulnerabilities and misconfigurations
3. Continuous Security Monitoring
Security isn't a point-in-time activity but requires ongoing vigilance across environments:
- Runtime application protection: Detect and block attacks in real-time
- Security information and event management (SIEM): Aggregate and analyze security data
- Behavioral analysis: Identify anomalous patterns that may indicate breaches
- Automated vulnerability scanning: Continuously check for new vulnerabilities in deployed applications
4. Embrace Collaborative Responsibility
DevSecOps isn't just about tools—it's a cultural transformation that makes security everyone's responsibility:
- Security champions: Embed security-minded individuals within development teams
- Cross-functional teams: Include security professionals in agile processes
- Blameless post-mortems: Focus on improving processes rather than punishing individuals
- Shared metrics: Align security, operations, and development on common goals
Implementing Security Controls in CI/CD Pipelines
A mature DevSecOps pipeline integrates security at each stage of the software delivery lifecycle:

Comprehensive security controls integrated throughout the CI/CD pipeline
Code Stage
The first line of defense is securing the code itself:
# Example pre-commit hook configuration (package.json)
{
"husky": {
"hooks": {
"pre-commit": "lint-staged && npm run security-scan"
}
},
"lint-staged": {
"*.js": ["eslint --fix", "git add"],
"*.{js,json,css,md}": ["prettier --write", "git add"]
},
"scripts": {
"security-scan": "npm audit && snyk test"
}
}
- Secrets detection: Prevent credentials from being committed to source control
- Code quality tools: Enforce security best practices through linting
- SAST: Identify vulnerabilities in custom code
- SCA: Check dependencies for known vulnerabilities
Build Stage
As code is compiled and packaged, additional security checks validate the artifacts:
- Artifact signing: Ensure integrity of build outputs
- Container scanning: Check container images for vulnerabilities and misconfigurations
- License compliance: Verify that all included components meet legal requirements
# Example Dockerfile with security best practices
FROM alpine:3.14 AS builder
# Add non-root user
RUN addgroup -S appgroup && adduser -S appuser -G appgroup
# Use multi-stage builds to reduce attack surface
WORKDIR /app
COPY --chown=appuser:appgroup . .
RUN pip install --no-cache-dir -r requirements.txt
# Remove unnecessary tools
FROM alpine:3.14
COPY --from=builder /app /app
USER appuser
ENTRYPOINT ["python", "/app/main.py"]
Test Stage
Dynamic testing reveals vulnerabilities that emerge from the application's runtime behavior:
- DAST: Automated security testing against running applications
- API security testing: Verify that APIs enforce proper authentication and authorization
- Fuzz testing: Send malformed inputs to discover edge case vulnerabilities
Deployment Stage
Infrastructure security is as critical as application security:
- Infrastructure as Code scanning: Validate security of Terraform, CloudFormation, or other IaC templates
- Compliance checks: Ensure all deployments meet regulatory requirements
- Immutable infrastructure: Prevent drift and unauthorized changes
# Example Terraform security best practices
resource "aws_s3_bucket" "data" {
bucket = "example-secure-bucket"
# Enable encryption
server_side_encryption_configuration {
rule {
apply_server_side_encryption_by_default {
sse_algorithm = "AES256"
}
}
}
# Block public access
block_public_acls = true
block_public_policy = true
ignore_public_acls = true
restrict_public_buckets = true
# Enable access logging
logging {
target_bucket = aws_s3_bucket.logs.id
target_prefix = "log/data-bucket/"
}
}
Runtime Stage
Continuous monitoring detects and responds to threats in production:
- Runtime application self-protection (RASP): Block attacks in real-time
- Web application firewall (WAF): Filter malicious traffic
- Continuous vulnerability scanning: Identify new vulnerabilities as they emerge
- Anomaly detection: Spot unusual patterns that may indicate compromise
Essential DevSecOps Tools and Frameworks
Building an effective DevSecOps toolchain requires integrating security capabilities across the development lifecycle:
SAST Tools
- SonarQube: Multi-language code quality and security scanner
- Checkmarx: Enterprise-grade static analysis
- Snyk Code: Developer-friendly SAST with quick feedback loops
- Semgrep: Lightweight, customizable static analysis
SCA Tools
- Snyk Open Source: Dependency vulnerability scanning with automatic fix PRs
- OWASP Dependency-Check: Open-source component analyzer
- WhiteSource: Comprehensive open source security and license compliance
- Sonatype Nexus IQ: Advanced component lifecycle management
DAST Tools
- OWASP ZAP: Open-source web application security scanner
- Burp Suite: Commercial web vulnerability scanner
- Acunetix: Automated web vulnerability scanning
- Synopsys Tinfoil: API and web application security testing
IaC Security
- Checkov: Static analysis for infrastructure code
- Terrascan: Detect compliance and security violations across IaC
- tfsec: Terraform-specific security scanner
- Bridgecrew: Cloud infrastructure security platform
Container Security
- Trivy: Comprehensive container vulnerability scanner
- Clair: Open-source container vulnerability analysis
- Aqua Security: Full lifecycle container security platform
- Sysdig Secure: Runtime container security and compliance
Secret Management
- HashiCorp Vault: Secrets management and protection
- AWS Secrets Manager: Cloud-based secrets management
- GitGuardian: Secrets detection and remediation
- Gitleaks: Git repository secrets scanner
Building a DevSecOps Culture
Tools alone don't create DevSecOps—cultural transformation is equally important:
From Gatekeeping to Enablement
Traditional security teams often acted as blockers, but DevSecOps demands a different approach:
- Self-service security: Provide tools and guidance that developers can use independently
- Security as code: Express security requirements as code that can be version-controlled and tested
- Prioritization frameworks: Help teams focus on the most critical issues first
- Developer-friendly reporting: Contextual, actionable security findings that make sense to developers
Building Security Champions Networks
Scale security expertise through a network of embedded champions:
- Identify security-minded developers: Look for engineers with interest in security
- Specialized training: Provide deeper security education for champions
- Recognition programs: Reward security contributions
- Regular knowledge sharing: Create forums for champions to exchange techniques
Measuring DevSecOps Success
Effective metrics help track security progress without hindering delivery:
- Mean time to remediate (MTTR): Average time to fix discovered vulnerabilities
- Security debt: Volume of known unaddressed security issues
- Automated test coverage: Percentage of code covered by security testing
- Deployment frequency: Ability to maintain delivery pace with security controls
- Failed security checks: Rate of builds failing due to security issues
Metric | Poor | Average | Excellent |
---|---|---|---|
MTTR (Critical Vulns) | > 30 days | 7-30 days | < 7 days |
Security Coverage | < 50% | 50-80% | > 80% |
Failed Security Checks | > 30% | 10-30% | < 10% |
DevSecOps in Regulated Industries
Organizations in regulated industries face additional challenges with DevSecOps implementation:
Compliance as Code
Automated compliance verification streamlines audits and reduces manual effort:
- Policy as code: Express compliance requirements as testable code
- Automated evidence collection: Generate audit artifacts automatically
- Continuous compliance monitoring: Verify adherence to regulations in real-time
- Immutable audit trails: Maintain tamper-proof records of security activities
Industry-Specific Considerations
Different industries have unique DevSecOps requirements:
- Financial Services: Focus on data protection, fraud prevention, and resilience
- Healthcare: Prioritize patient data privacy and medical device security
- Government: Emphasize supply chain security and classified data protection
- Retail: Balance customer data protection with frictionless experiences
Overcoming DevSecOps Implementation Challenges
Based on my experience, these are the most common roadblocks to DevSecOps adoption and how to address them:
Tool Fragmentation
The proliferation of security tools creates integration challenges:
- Consolidate tooling: Choose platforms that cover multiple security aspects
- Standardize outputs: Normalize findings into consistent formats
- Security orchestration: Use platforms that coordinate across tools
- API-first selection: Prioritize tools with robust integration capabilities
False Positives
Excessive false positives erode trust in security automation:
- Tuning and customization: Adjust tool settings for your specific environment
- Risk-based prioritization: Focus on high-confidence, high-impact findings first
- Contextual analysis: Correlate findings across tools to reduce noise
- Continuous improvement: Track and reduce false positive rates over time
Skills Gaps
DevSecOps requires a blend of security and development expertise:
- Cross-training programs: Build security skills in developers and development understanding in security teams
- Hands-on workshops: Practice security techniques in realistic scenarios
- Security certifications: Support relevant professional development
- Capture-the-flag exercises: Gamify security education
The Future of DevSecOps
The DevSecOps landscape continues to evolve with these emerging trends:
AI and Machine Learning
Intelligent security automation is transforming DevSecOps:
- Predictive vulnerability analysis: Anticipate security issues before they manifest
- Intelligent triage: Automatically prioritize security findings based on context
- Anomaly detection: Identify unusual patterns that indicate potential breaches
- Natural language policy interpretation: Translate compliance requirements into code automatically
Zero Trust Supply Chains
Advanced supply chain security measures are becoming essential:
- Software bill of materials (SBOM): Maintain detailed inventories of all components
- Provenance verification: Cryptographically verify the origin of all code
- Chain of custody: Track all changes to artifacts throughout the pipeline
- Reproducible builds: Ensure build outputs are identical given the same inputs
Cloud-Native Security
Security approaches specifically designed for cloud environments:
- Service mesh security: Control service-to-service communication
- Serverless security: Protect function-based architectures
- Kubernetes-native security controls: Leverage platform capabilities for enforcement
- Identity-aware proxies: Implement zero trust networking
Conclusion
DevSecOps represents a fundamental shift in how organizations approach security—moving from a bottleneck to an enabler of rapid, secure delivery. By integrating security throughout the software development lifecycle and fostering a culture of shared responsibility, teams can achieve both velocity and safety.
While implementing DevSecOps requires significant organizational change and tooling investments, the return on investment is substantial: reduced security incidents, lower remediation costs, improved compliance posture, and the ability to deliver secure software at the pace the business demands.
As cyber threats grow more sophisticated and regulations more stringent, DevSecOps isn't just a competitive advantage—it's becoming a baseline requirement for responsible software development. Organizations that successfully implement these practices will be better positioned to navigate the complex security landscape while continuing to innovate rapidly.
Related Articles
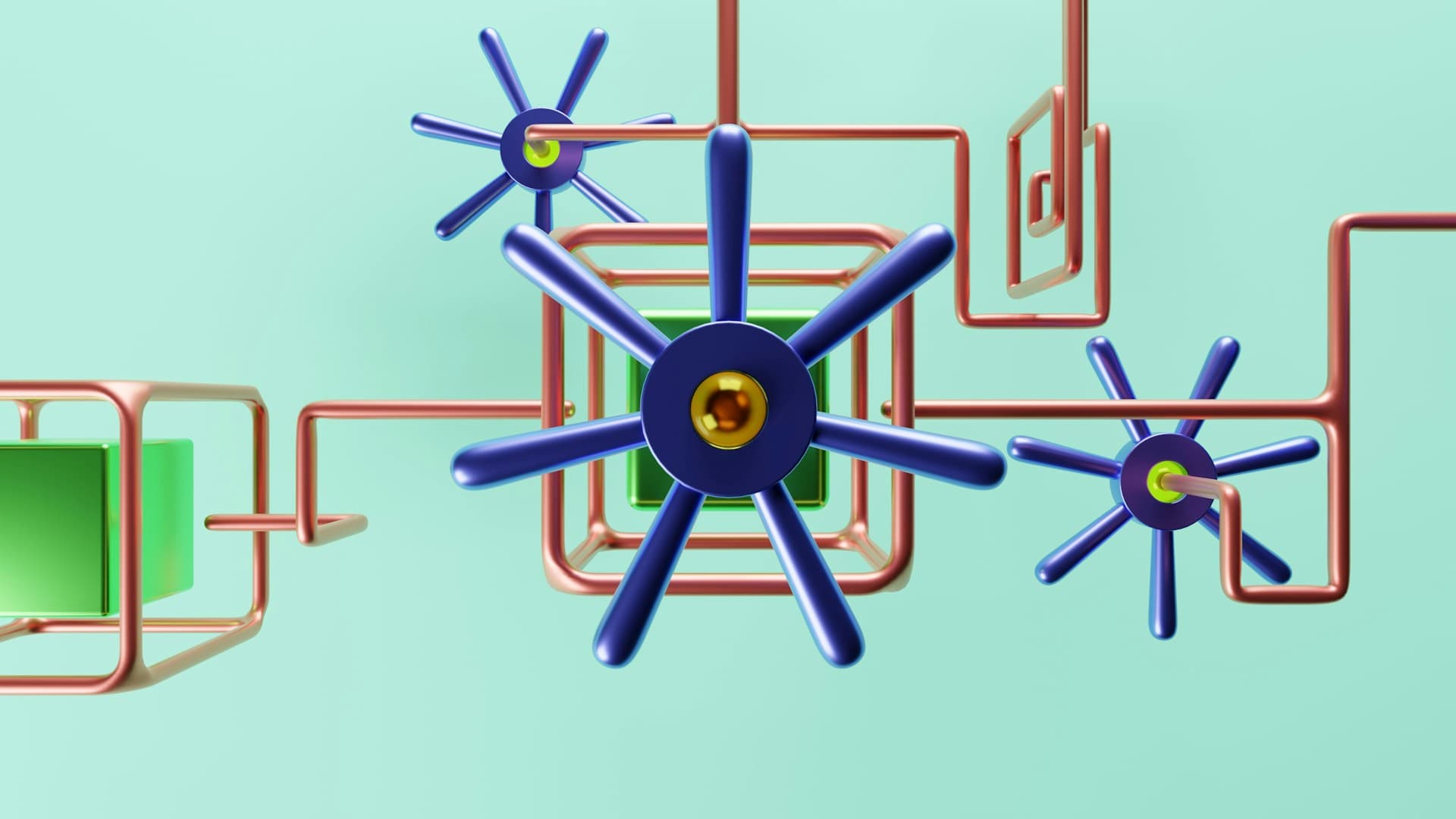
CI/CD Pipeline Best Practices for Modern Development Teams
Continuous Integration and Continuous Delivery (CI/CD) are essential practices for modern software development. Learn the best practices for implementing effective CI/CD pipelines.
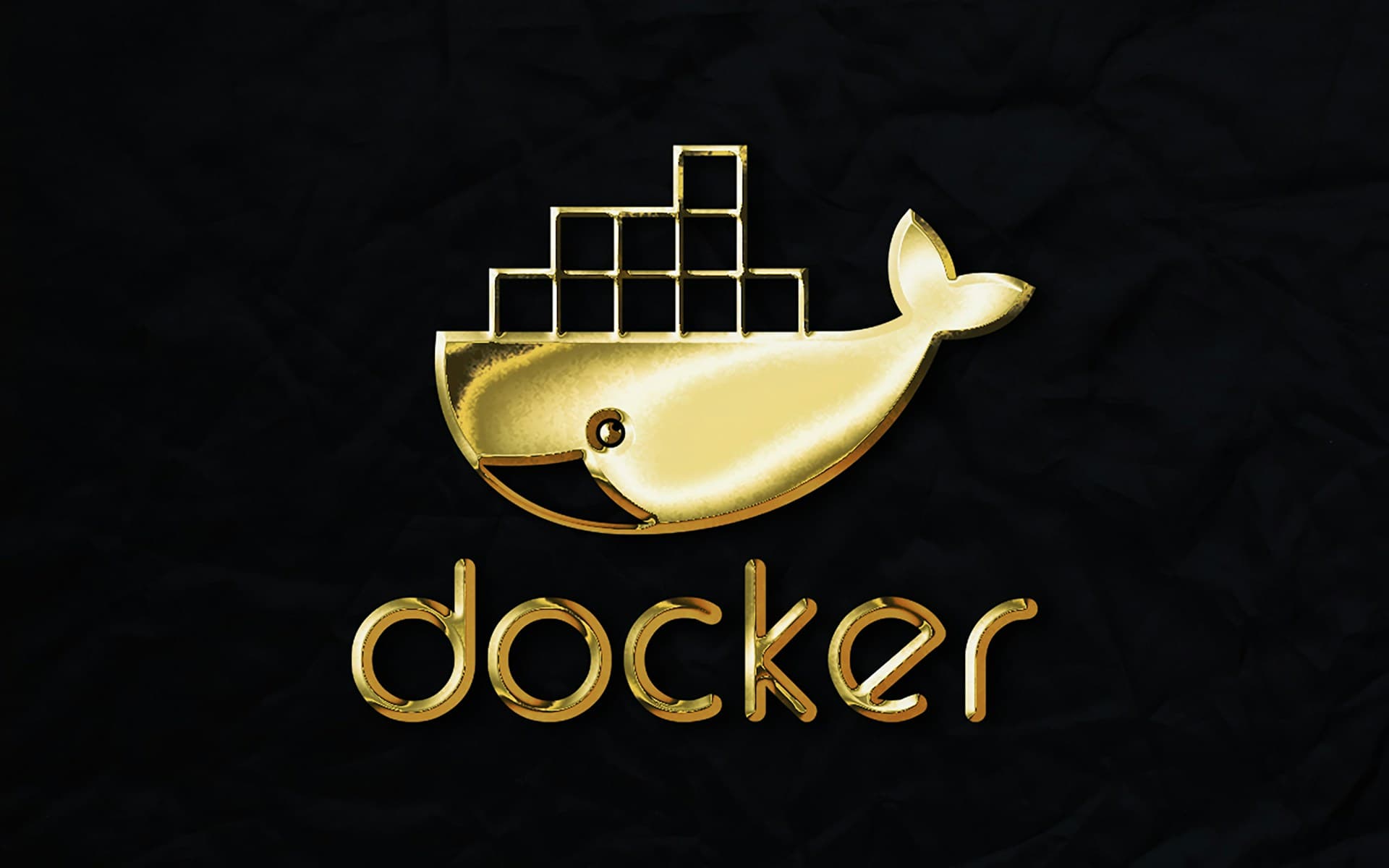
Enterprise-Grade Docker: Production Hardening and Optimization Techniques
Master production-ready containerization with battle-tested Docker best practices. Learn advanced security hardening, performance optimization, resource governance, and orchestration strategies derived from real-world enterprise deployments.
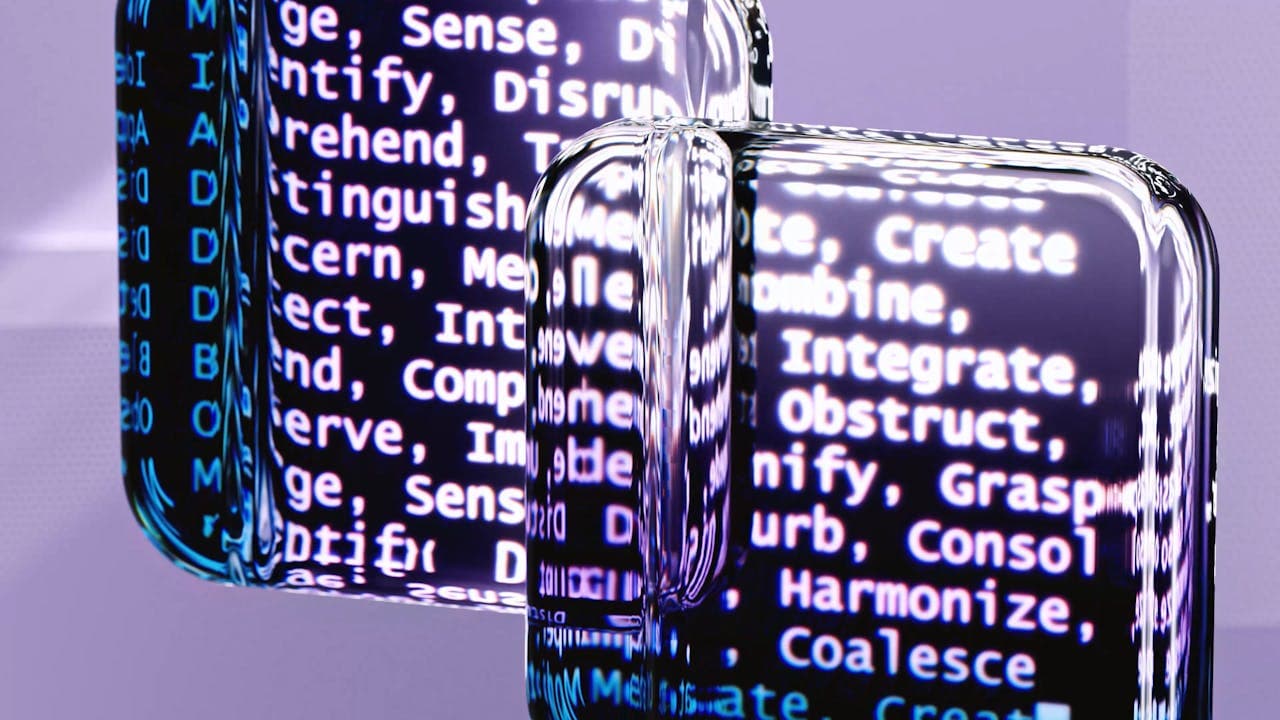
Enterprise Infrastructure as Code: Architecting Cloud-Native Platforms with Terraform
Discover how to implement infrastructure as code (IaC) at scale with Terraform. Learn battle-tested patterns for managing complex, multi-cloud environments, state management strategies, and CI/CD pipeline integration from a senior platform engineering perspective.